Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial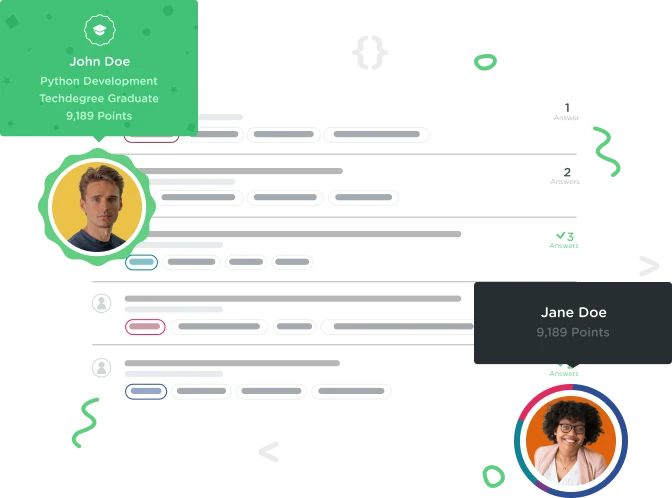

James Thompson
2,475 PointsMy solution to the challenge.
Here was my solution to the challenge. At first I was not for sure how to actually use the question part of the array so I ignored it with my implementation. Did anyone else try something similar?
// 1. Create a multidimensional array to hold quiz questions and answers const quiz_game = [ ["How many letters are in the color red?", "three"], ["How many letters are in the color orange?", "six"], ["How many letters are in the color blue?", "four"]
];
// 2. Store the number of questions answered correctly
/*
- Use a loop to cycle through each question
- Present each question to the user
- Compare the user's response to answer in the array
- If the response matches the answer, the number of correctly answered questions increments by 1 */
let message; // initializing a variable to write a message. let correct_answer = 0; // initializing a variable to hold the correct amount of numbers.
answer_1 = prompt("How many letters are in the color red?"); answer_2 = prompt("How many letters are in the color orange?"); answer_3 = prompt("How many letters are in the color blue?");
for (let i = 0; i < 1; i++) {
if (!answer_1 || !answer_2 || !answer_3) {
message = console.log("Please input answers to the quiz.");
break;
}
if (answer_1 === quiz_game[0][1]) {
correct_answer += 1;
} if(answer_2 === quiz_game[1][1]) { correct_answer += 1;
} if(answer_3 === quiz_game [2][1]) { correct_answer += 1;
}
}
message = console.log(You got ${correct_answer} questions correct!!
);
// 4. Display the number of correct answers to the user
1 Answer

Karla Judith Najera Vera
4,415 PointsThis is my solution
const quiz = [ ['How many planets are in the Solar System', '8'], ['Which is the largest planet in the Solar System', 'JUPITER'], ['What planet is after Earth?', 'MARS'], ['How many continents are there?', '7'], ['How many legs does an insect have?','6'], ['What year was JavaScript created?', '1995']
];
// 2. Store the number of questions answered correctly
let correctAnswers = 0
//3. Use a loop to cycle through each question // - Present each question to the user // - Compare the user's response to answer in the array // - If the response matches the answer, the number of correctly // answered questions increments by 1
for (let i = 0; i < quiz.length; i++ ) {
const askedQuestions = ${quiz[i][0]}
;
const quizAnswers = ${quiz[i][1]}
;
const response = prompt(${askedQuestions}
);
if (response.toUpperCase() === quizAnswers) { correctAnswers++;
}
}
//4. Display the number of correct answers to the user
document.querySelector('main').innerHTML = <h1>You have ${correctAnswers} correct answers!</h1>
;
Simon Coates
8,177 PointsSimon Coates
8,177 PointsYou should be able to see what some other people were trying to do via the questions for that video. Even if they got stuck it might give you an insight into approaches taken. I don't think I've done that video series, but I decided to throw a (poorly tested) demo together. I came up with:
You seemed to be trying to do validation despite this not fitting well with your for loop. To do validation, i added a test on answer and manually performed the for loop's increment. If there's no answer, it continues and never gets to the i++ line to proceed to the next question.