Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial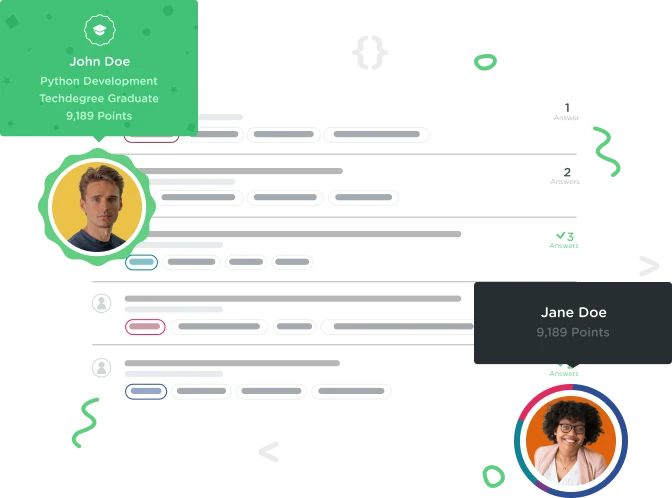

Fidel Severino
3,514 PointsMy solution to the JS Refactor Challenge w/ comments
Just posting my solution to this challenge. I thought this has been one of the better challenges on this track so far. Looking at other people's solutions is fun since it shows different ways to accomplish the same result.
The comments feel too verbose, but that was the goal because I wanted to make sure they were very clear for those who are new to JS/programming like me. I assume with more time and practice my comments will become more succinct.
var html = '',
rgbColor;
/*
function that creates a random combination of
red, blue and green values and then stores them
in the rgbColor variable
*/
function rgbCreator() {
var red = Math.floor(Math.random() * 256 ), // creates random shade of red
green = Math.floor(Math.random() * 256 ), // random shade of green
blue = Math.floor(Math.random() * 256 ); // random shade of blue
rgbColor = 'rgb(' + red + ',' + green + ',' + blue + ')';
}
/*
for loop which calls the rgbCreator() function to
create a new rgb value each time it loops. Each time
it adds a new div to the html variable with the
randomly generated color
*/
for (var i = 0; i < 10; i++) {
rgbCreator();
html += '<div style="background-color:' + rgbColor + '"></div>';
}
document.write(html);
3 Answers
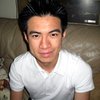
jason chan
31,009 PointsGoodjob man it's clean, efficient, and poetic. Beautiful code.
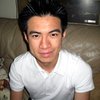
jason chan
31,009 PointsKeep up the good work man. Good code! Cheers ;)
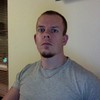
James Tighe
2,113 PointsThat is a really concise bit of coding. Pretty much what I got to. Nice commenting as well. I find it really helps.
Fidel Severino
3,514 PointsFidel Severino
3,514 PointsThanks a lot, Jason. Nice to hear it's clean and efficient code. Don't know about poetic :P
Kristian Terziev
28,449 PointsKristian Terziev
28,449 PointsIt is indeed quite poetic I have to say.