Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial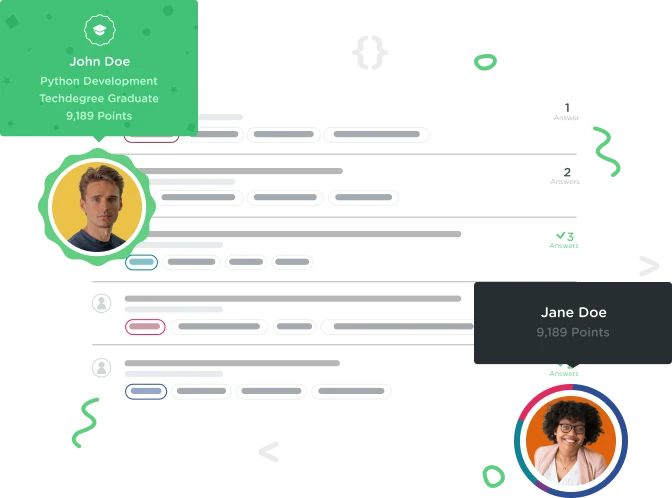
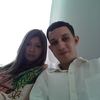
Raul Vega
Courses Plus Student 3,803 PointsMy solution to the Quiz Challenge Pt. 2 (Feedback appreciated)
var correctAnswers = [];
var incorrectAnswers = [];
var questions = [
['Which is the biggest planet on our galaxy?', 'jupiter'],
['Which is the second planet from the sun?', 'venus'],
['To which planet we refer to as the "Red Planet"?', 'mars']
];
//The id paramenter was added in case we need to use this function in other elements of the page.
function print(id,message) {
var outputDiv = document.getElementById(id);
outputDiv.innerHTML = message;
}
for ( var i = 0; i < questions.length; i+= 1 ){
var userInput = prompt(questions[i][0]);
userInput = userInput.toLowerCase();
if (userInput === questions[i][1]){
alert("Correct!");
correctAnswers.push(questions[i][0]);
}else{
alert("Incorrect!");
incorrectAnswers.push(questions[i][0]);
}
}
var html = "<h1>Results</h1>";
html+= "<i>You answered "+correctAnswers.length+" out of "+questions.length+" questions correctly!</i>";
html+= "<h2>Correct Answers</h2>";
if (correctAnswers.length > 0){
html+= "<ol><li>";
html+= correctAnswers.join("</li><li>");
html+= "</li></ol>";
}else{
html+= "<i>You did not answered correctly any of the questions.</i>";
}
if (incorrectAnswers.length > 0){
html+= "<h2>Incorrect Answers</h2>";
html+= "<ol><li>";
html+= incorrectAnswers.join("</li><li>");
html+= "</li></ol>";
}
//Print the html to the element containing the 'output' id.
print('output',html);
1 Answer
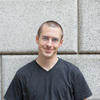
Nathan Brenner
35,844 PointsFirst, our code on lines 30 to 33 are very similar to 37 to 41, which means you probably have an opportunity to write a function where you can pass in an array as an argument, which would be the correct answers array the first time you call that method, and the incorrect answers the second time you call it.
Next, I don't think 'join' will return what you think it will.
For example: console.log([1, 2, 3].join());
returns "1,2,3"
What you want is to loop through the array and print each within a separate list item, which you can do like this:
for(var i = 0; i<3; i++){ document.write("<li>" + i + "</li>")
I would imagine you went on to the next video to see his solution, but can you connect these two suggestions to revise your code?