Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial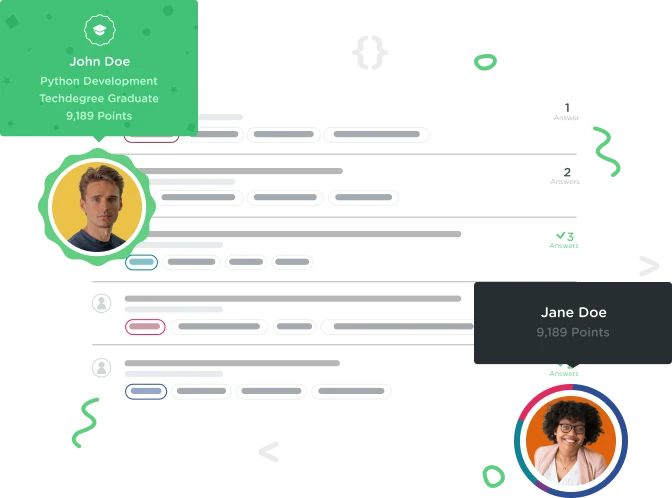

Michael Lambert
6,286 PointsMy solution to the quiz task
// generate random number
function randomNumber(upper, lower) {
return Math.floor(Math.random() * (upper - lower + 1)) + lower;
}
// get inputs from user and display the result
function getNumbers() {
var num1 = parseInt(prompt('Enter first number'));
var num2 = parseInt(prompt('Enter second number'));
var upperNum = Math.max(num1, num2);
var lowerNum = Math.min(num1, num2);
console.log(randomNumber(upperNum, lowerNum));
}
// run it 5 times
getNumbers();
getNumbers();
getNumbers();
getNumbers();
getNumbers();
I havn't checked it extensively but it seems to be working as expected. Is there anything i can do to streamline the code while maintaning the same functionality?
3 Answers
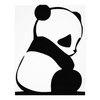
Peter Quin
3,203 PointsMaybe you could add a for loop instead of the five calls:
for (i = 0; i < 5; i++) {
getNumbers();
}
removes a few lines
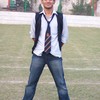
Shivang Chaturvedi
2,600 PointsCheck this out

Michael Lambert
6,286 PointsI had considered using conditionals for checking min and max but i ended up trying out the Math.max & Math.min which i stumbled across earlier in the week but didn't have cause to use them at the time. Thanks for the example though :)

Tyler Corum
3,514 PointsAt the end of your code, Shivang, you don't need to create a variable called numArgument, nothing is being returned anyway. Just call the function...
randomNumber(num1, num2);
Keep up the good work.

Tyler Corum
3,514 PointsVery readable, great job. I like your descriptive variable and function names!
Michael Lambert
6,286 PointsMichael Lambert
6,286 PointsI haven't got that far in the course yet where they cover loops but it looks simple enough to understand so thanks for the introduction to using them :). I've implemented your code into it now and set it to 10. Saves a whole bunch of repeated code.