Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial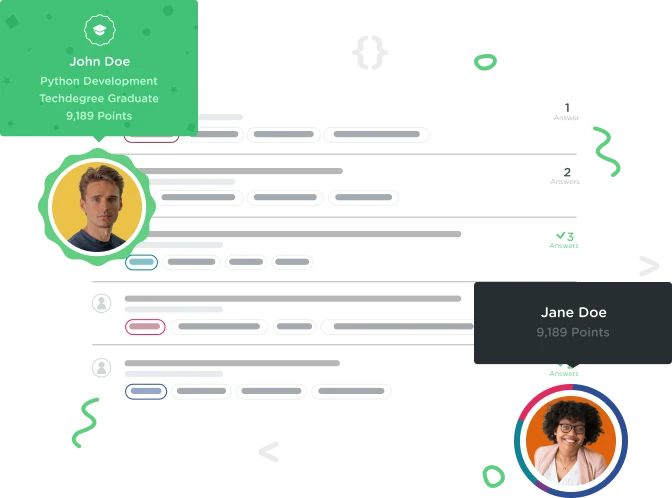

T. Gontarek
7,030 PointsMy solution to the Random Number Challenge!
// Collect input from a user
let userInput = prompt("Enter a number into the text box bellow.");
// Convert the input to a number
userInput = parseInt(userInput);
// Use Math.random() and the user's number to generate a random number
let randomNumber = Math.floor(Math.random()* userInput)+1;S
// Create a message displaying the random number
let message = `The random number generated from ${userInput} is ${randomNumber}.`
console.log(message);
5 Answers
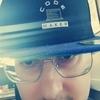
Magnus Martin
44,123 PointsHere is mine.
// Collect input from a user
const input = prompt("Enter a number into the text box bellow.");
// Convert the input to a number
inputNumber = parseInt(input);
// Use Math.random() and the user's number to generate a random number
const randomNumber = Math.floor(Math.random() * inputNumber) + 1;
// Create a message displaying the random number
const answerWrapper = document.createElement("div");
const answer = document.createTextNode(randomNumber);
answerWrapper.appendChild(answer);
document.getElementsByTagName('main')[0].appendChild(answerWrapper);
Any improvements or suggestions?
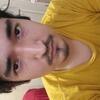
Adrian Lemus
Full Stack JavaScript Techdegree Student 1,112 PointsIt's so fascinating seeing everyones input. Mine seems a bit long in comparison to everyone else's.
// Collect input from a user
const userAnswer = prompt('Please Provide a number.');
const main = document.querySelector('main');
// Convert the input to a number
parseInt(userAnswer);
console.log(userAnswer);
// Use Math.random() and the user's number to generate a random number
let firstGenerator = Math.floor(Math.random() * userAnswer ) + 1;
alert(`${firstGenerator} is a number between 1 and ${userAnswer}.`);
console.log(firstGenerator);
// Create a message displaying the random number
main.innerHTML = (`<p><stong>${firstGenerator }</strong> is a number between 1 and <strong>${userAnswer}.</strong></p>`);
console.log(`${firstGenerator} is a number between 1 and ${userAnswer}.`);
Any input, suggestions or comments for my code are appreciated. Thanks!

kevin hudson
Courses Plus Student 11,987 Pointsconst main = document.querySelector('main');
// Collect input from a user
let inputNum = prompt('Please select a number to output a random between 1 and that number:')
// Convert the input to a number
inputNum = +inputNum;
// Use Math.random() and the user's number to generate a random number
const output = Math.floor(Math.random()* inputNum) + 1;
// Create a message displaying the random number
main.innerHTML = `<p>${output} is a random number between 1 and ${inputNum}.</p>`
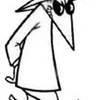
Gerhard Driedger
7,486 PointsI appreciate seeing different people's methods so here is mine
// Collect input from a user
const picker = prompt('Please pick a number');
// Convert the input to a number
const dividend = +picker;
// Use Math.random() and the user's number to generate a random number
const divisor = Math.round(Math.random()*10);
const quotient = dividend / divisor;
// Create a message displaying the random number
alert(`${dividend} divided by ${divisor} is ${quotient}`);

Ken Del Valle
976 Points// Collect input from a user
let userInput = prompt("Heyo! Type in a number.");
// Convert the input to a number
let newNumber = parseInt(userInput);
// Use Math.random() and the user's number to generate a random number
let ultimate = Math.random() * newNumber + 1;
// Create a message displaying the random number
alert(`Your lucky number is ${ultimate}. Don't wear it out!`);