Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial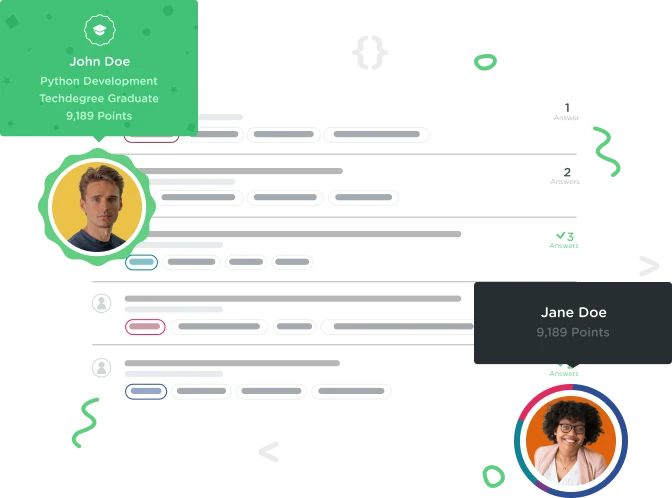

Marco Fregoso
223 PointsMy solution to this
This is my solution, I tried to keep my code as "DRY" as possible
// declare program variables
// announce the program
alert("Let's do some math!");
// collect numeric input
var num1 = askForParsedNumber();
var num2 = askForParsedNumber();
// build an HTML message
var message = "<h1>Math with the numbers " + num1 + " and " + num2 + "</h1>";
message += num1 + " + " + num2 + " = " + (num1 + num2);
message += "<br>";
message += num1 + " * " + num2 + " = " + (num1 * num2);
message += "<br>";
message += num1 + " / " + num2 + " = " + (num1 / num2);
message += "<br>";
message += num1 + " - " + num2 + " = " + (num1 - num2);
// write message to web page
document.write(message);
function askForParsedNumber() {
do {
var number = typeNumber();
number = parseFloat(number);
if(isNaN(number)) {
alert("Value entered is not a number!");
continue;
} else if(number === 0) {
alert("0 is not a allowed number please choose another one");
continue;
} else {
break;
}
} while(true)
return number;
}
function typeNumber() {
var promptValue = prompt("Please type a number");
return promptValue;
}
Let me know what you guys think
3 Answers

James Anwyl
Full Stack JavaScript Techdegree Graduate 49,960 PointsNice work, looks pretty dry to me!
I would suggest adding some comments explaining what the functions are doing, will help other people as well as yourself if you go back to it later. Would also suggest wrapping the messages inside p tags or maybe a list.
Hope this helps :)
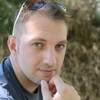
Doron Geyer
Full Stack JavaScript Techdegree Student 13,897 Pointshavent done functions yet but here what I did
// declare program variables
var num1;
var num2;
var message;
// announce the program
alert("Let's do some math!");
// collect numeric input
num1 = prompt("Please type a number");
num1 = parseFloat(num1);
num2 = prompt("Please type another number");
num2 = parseFloat(num2);
if(num2 === 0){
alert("you cannot divide by 0 please enter a new number");
num2 = parseFloat(prompt("please provide us with a non 0 number."));
}
if(((isNaN(num1)) || (isNaN(num2))) || num2===0){
alert("At least one of the values you typed is not a number or = 0. Reload and try again.");
}else {
// build an HTML message
message = "<h1>Math with the numbers " + num1 + " and " + num2 + "</h1>";
message += num1 + " + " + num2 + " = " + (num1 + num2);
message += "<br>";
message += num1 + " * " + num2 + " = " + (num1 * num2);
message += "<br>";
message += num1 + " / " + num2 + " = " + (num1 / num2);
message += "<br>";
message += num1 + " - " + num2 + " = " + (num1 - num2);
// write message to web page
document.write(message);
}

NICOLE REBENTISCH
3,612 PointsI wish I had done mine the way shown by the instructor but I just didn't see it! Mine gets the job done but less elegantly. Here's what I wrote:
// declare program variables var num1; var num2; var failMessage= 0; var message;
// announce the program alert("Let's do some math!");
// collect numeric input num1 = prompt("Please type a number"); num1 = parseFloat(num1);
if (isNaN(num1)|| num1 === NaN ){ alert( "At least one of the values you typed is not a number. Reload and try again."); failMessage = 1; }
else if (num1 !== NaN) { num2 = prompt("Please type another number"); num2 = parseFloat(num2); }
else if ( (isNaN(num2) || num2 === NaN) && (num1 !== NaN)){ alert( "At least one of the values you typed is not a number. Reload and try again."); failMessage = 1; }
else if (num2 === 0){ alert("The second number is 0. You can't divide by zero. Reload and try again."); }
// build an HTML message
message = <h1>Math with the numbers ${num1} and ${num2}</h1>
${num1} + ${num2} = ${num1 + num2}
<br>
${num1} * ${num2} = ${num1 * num2}
<br>
${num1} / ${num2} = ${num1 / num2}
<br>
${num1} - ${num2} = ${num1 - num2}
;
// write message to web page
if (failMessage === 0){ document.write(message); }