Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial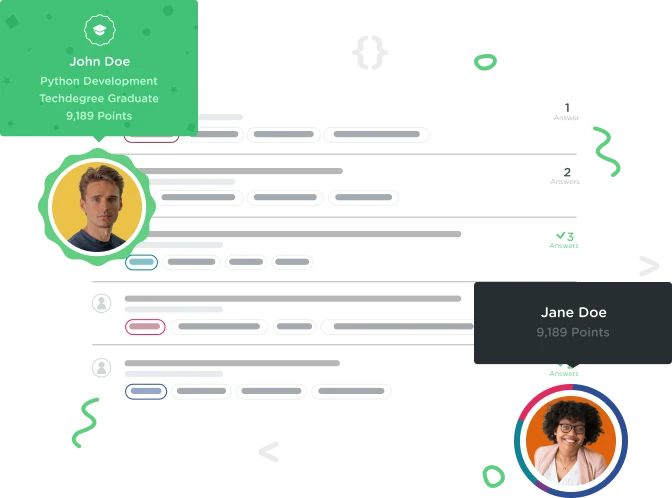

franckmc
9,095 PointsMy solution vs. modular approach
Hello everyone, I'd like to know why is it better to use more function (modular approach) when programming ? Because the solution I found still works, follows the DRY principle and uses very few lines.
Thank you
var html = '', rgbColor;
for(var i=0; i < 10; i++){
rgbColor = 'rgb(' + randomizer() + ',' + randomizer() + ',' + randomizer() + ')';
html += '<div style="background-color:' + rgbColor + '"></div>';
}
function randomizer(){
return Math.floor(Math.random()*256);
}
document.write(html);
2 Answers
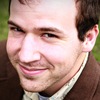
Daniel Hurd
12,987 Points"why is it better to use more function (modular approach) when programming ?" --> great question! It made me think about what we've learned so far, and here's what I've come up with:
Because functions offer you concise, reusable code.
Your code here is sufficient:
rgbColor = 'rgb(' + randomizer() + ',' + randomizer() + ',' + randomizer() + ')';
But, creating an additional function (which is initially longer and adds more lines) allows us to capture your three randomizer()
functions into one:
rgbColor = randomColor();
While the randomColor()
function is longer code at the outset, we can now use randomColor()
wherever we want! If we wanted to reuse your code we'd need to output randomizer()
three times plus use the concatenated rgb string just like you have above. In the end, then, your code would not actually align with the DRY principle, whereas the additional function randomColor()
would.
Does that make sense? And what do you think? Thanks for your question - it helped me think through all of this!

franckmc
9,095 PointsThanks for your enlightment Daniel Hurd !
I guess I didn't see that using my randomizer() function three times was not DRY-friendly. Following the modular approach, I just made an adjustement and created this function:
function color(){
var r, g, b;
r = randomizer(), g = randomizer(), b = randomizer();
var output = 'rgb(' + r + ',' + g + ',' + b + ')';
return output;
}
It makes more sense, since I just need to call color() for my variable rgbColor.
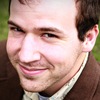
Daniel Hurd
12,987 PointsNice. Thanks for sharing!
Nicholas Woods
6,260 PointsNicholas Woods
6,260 PointsI was wondering the same thing. I guess it keeps the loops tidier, and the function is only used when it is called.