Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial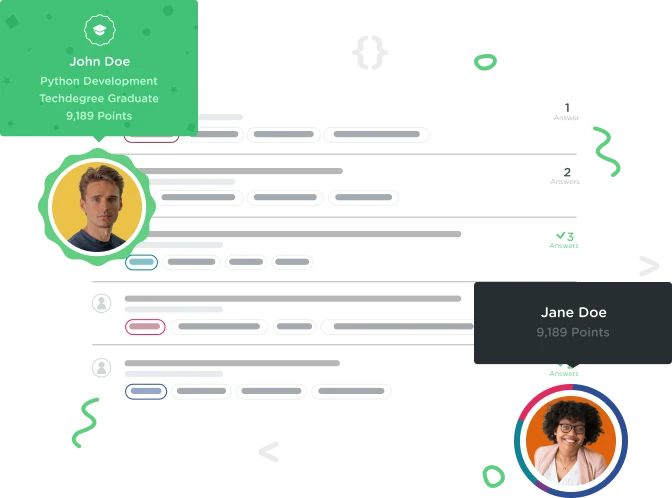

Jesse Benedict
Courses Plus Student 4,338 PointsMy solution w/ while loop used to keep asking the user for a valid input
// Collect input from a user
let randLow = prompt("Input a number and we will provide a random number between this number and another number");
let randHigh = prompt(`Input a number and we will provide a random number between ${randLow} and this number`);
// Check if user inputs are integers
if(isNaN(+randLow) || isNaN(+randHigh)){
/*
while loop to check if any of the inputs are invalid
isNaN method checks if a variable is NaN
If either one is not an integer, it will keep on asking the user for one.
*/
while(isNaN(+randLow) || isNaN(+randHigh)){
// if low input is not an integer
if(isNaN(+randLow)) {
document.querySelector('main').innerHTML = `<h2>${randLow} is not a number, try again.</h2>`;
randLow = prompt("Input a number and we will provide a random number between this number and another number");
}
// if high input is not an integer
if(isNaN(+randHigh)) {
document.querySelector('main').innerHTML = `<h2>${randHigh} is not a number, try again.</h2>`;
randHigh = prompt(`Input a number and we will provide a random number between ${randLow} and this number`);
}
}
// if both are now integers
if(!(isNaN(+randLow) || isNaN(+randHigh))) {
// Use Math.random() and the user's numbers to generate a random number
let randNum = Math.floor(Math.random() * (+randHigh - +randLow + 1)) + +randLow
// Create a message displaying the random number
document.querySelector('main').innerHTML = `<h2>${randNum} is a number between ${randLow} & ${randHigh}!</h2>`;
}
} else { // if both are right out of the bat
// Use Math.random() and the user's number to generate a random number
let randNum = Math.floor(Math.random() * (+randHigh - +randLow + 1)) + +randLow
// Create a message displaying the random number
document.querySelector('main').innerHTML = `<h2>${randNum} is a number between ${randLow} & ${randHigh}!</h2>`;
}