Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial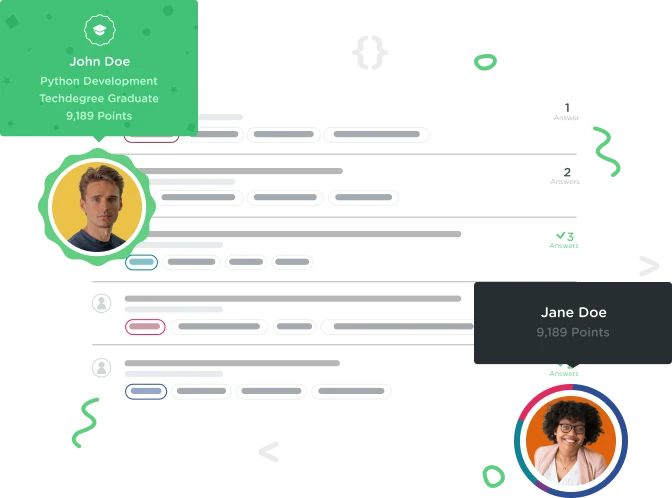
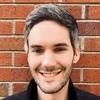
Jesse Vorvick
6,047 PointsMy solution was only the same, but with a minor difference that lead to some useful info
I had to play around with this to get it to work, but when I did, I was thrilled to see it was similar to Dave's method in the solution. However, there is one key difference with my code that may provide some useful information.
// declare program variables
var num1;
var num2;
var message;
// announce the program
alert("Let's do some math!");
// collect numeric input
num1 = prompt("Please type a number");
num1 = parseFloat(num1);
num2 = prompt("Please type another number");
num2 = parseFloat(num2);
// build an HTML message
message = "<h1>Math with the numbers " + num1 + " and " + num2 + "</h1>";
message += num1 + " + " + num2 + " = " + (num1 + num2);
message += "<br>";
message += num1 + " * " + num2 + " = " + (num1 * num2);
message += "<br>";
message += num1 + " / " + num2 + " = " + (num1 / num2);
message += "<br>";
message += num1 + " - " + num2 + " = " + (num1 - num2);
/*
check that num2 is not zero,
check that num1 and num2 are numbers,
otherwise write message to web page
*/
if ( num2 === 0) {
alert("The second number is 0. You can't divide by zero. Reload and try again.");
} else if ( isNaN(num1) || isNaN(num2) ){
alert("At least one of the values you typed is not a number. Reload and try again.");
} else {
document.write(message);
}
The difference is in the final "else" clause. At first, I had removed document.write from the bottom all together and stuck it with the rest of the conditional statements. The mistake I made was that because in the original structure of the challenge the HTML message came AFTER the conditional statements, I was left with broken code. I suppose this is because the program tried to write the message variable before it had any value stored to it.
So, as shown above, I simply switched the order. I put the HTML message BEFORE the conditional statements.
In looking at the solution video, I noticed that Dave had an even simpler solution: he put the HTML message entirely within the brackets of the "else" clause, leaving document.write at the bottom and everything more or less in its original order.
What do you guys think? Does either solution matter? Are there difficulties with either methods that could cause problems in the future with more advanced coding? Let me know your thoughts!
1 Answer
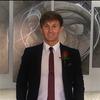
Liam Clarke
19,938 PointsHi Jesse
Its good that you logically see how the use of a method can make your conditions much more readable, this will put you in a good position for when you learn stuff like functions and and then more advanced topics like design patterns - This is a very good read and object oriented principles
However, the main difference in your example is, whether you write it to the document or not, behind the scenes that code is being run. Now that's not an issue here because it happens in milliseconds but lets say we took this a step further and we displayed the numbers and underneath a multiplication table, division table, subtraction table and addition table or something like that, string concats can really effect your programs optimization, check this stack answer.
In Dave's example, the string concat is created only when the condition is met, test this out yourself by opening the developer tools (Chrome - press F12) and add a breakpoint on your message string.
So without overwhelming you with detail, the way you would do this is to create functions and then pointing to them within the conditional statements, you essentially did that with the write method, you point to the message variable, so your definitely on the right track to understanding how to keep your code DRY and well strctured
Let me know if you'd like anymore information
Good Luck!
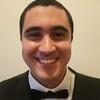
Lucas Guimarães
Front End Web Development Techdegree Student 3,900 PointsYou are right. I did the same and the worst thing is, I knew it but I just ignored it. But now I learned. Thank you so much for your explanation.
Dave StSomeWhere
19,870 PointsDave StSomeWhere
19,870 PointsI would be concerned that where you build your message you do math on num1 and num2 before verifying that they are numbers. I think it would be a good idea to be very careful when working with numbers and many languages will blow up when attempting to do number stuff on non numbers.
Kudos for investigating your own ideas - good train of thought...