Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial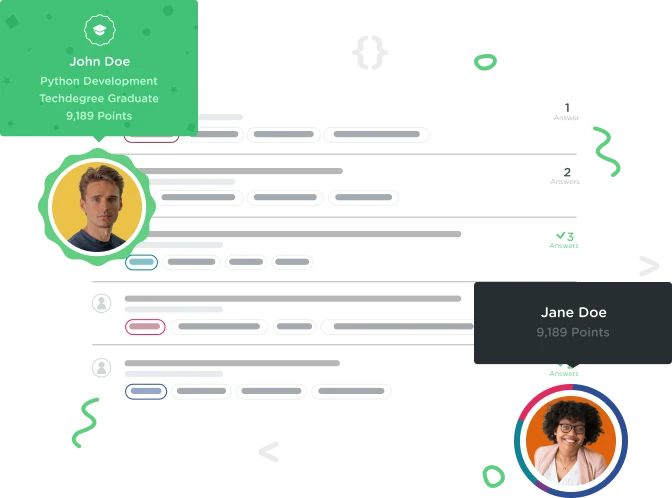

Bruce Kean
6,864 PointsMy solution (with code blocks) to final challenge. Any feedback would be really appreciated.
Here is my solution to the final challenge. It seems to work but I'm new to JavaScript so don't know if it's best practice or not. Any feedback would be really appreciated.
var students = [
{
name: 'Dave',
track: 'Front End Development',
achievements: 158,
points: 14730
},
{
name: 'Jody',
track: 'iOS Development with Swift',
achievements: '175',
points: '16375'
},
{
name: 'Jody',
track: 'PHP Development',
achievements: '55',
points: '2025'
},
{
name: 'John',
track: 'Learn WordPress',
achievements: '40',
points: '1950'
},
{
name: 'Trish',
track: 'Rails Development',
achievements: '5',
points: '350'
}
];
var message = '';
var student;
var search;
function print(message) {
var outputDiv = document.getElementById('output');
outputDiv.innerHTML = message;
}
function getReport( student ){
var report = '<h2>Student: ' + student.name + '</h2>';
report += '<p>Track: ' + student.track + '</p>';
report += '<p>Points: ' + student.points + '</p>';
report += '<p>Achievements: ' + student.achievements + '</p>';
return report;
}
while(true){
search = prompt("Search for a student by name or type 'quit' to exit");
if( search === null || search.toLowerCase() === 'quit' ){
break;
} else {
print("<h2>There is no student by that name</h2>");
}
for (var i = 0; i < students.length; i += 1) {
student = students[i];
if( search === student.name ) {
message += getReport( student );
print(message);
continue;
}
}
message = ' ';
}
6 Answers
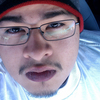
Chyno Deluxe
16,936 PointsGreat Job! My only suggestion would be to keep your student points and achievements consistent by keeping them as integers rather than strings of numbers.
var students = [
{
name: 'Dave',
track: 'Front End Development',
achievements: 158,
points: 14730
},
{
name: 'Jody',
track: 'iOS Development with Swift',
achievements: 175,
points: 16375
},
{
name: 'Jody',
track: 'PHP Development',
achievements: 55,
points: 2025
},
{
name: 'John',
track: 'Learn WordPress',
achievements: 40,
points: 1950
},
{
name: 'Trish',
track: 'Rails Development',
achievements: 5,
points: 350
}
];

Bruce Kean
6,864 PointsThanks for the feedback. Hadn't realised I'd done that. Thanks for pointing it out :)
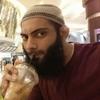
Muhammad Athar
4,004 PointsI am trying to understand how the continue keyword was able to help with the solution. Can you please explain the logic.

Bruce Kean
6,864 PointsI've just checked and it actually needn't be there. I tried a few different solutions before landing on this one so probably left it there from a previous attempt. The amended solution is;
var message;
var student;
var search;
function print(message) {
var outputDiv = document.getElementById('output');
outputDiv.innerHTML = message;
}
function getReport( student ){
var report = '<h2>Student: ' + student.name + '</h2>';
report += '<p>Track: ' + student.track + '</p>';
report += '<p>Points: ' + student.points + '</p>';
report += '<p>Achievements: ' + student.achievements + '</p>';
return report;
}
while(true){
search = prompt("Search for a student by name or type 'quit' to exit");
message = ' ';
if( search === null || search.toLowerCase() === 'quit' ){
break;
} else {
print("<h2>There is no student by that name</h2>");
}
for (var i = 0; i < students.length; i += 1) {
student = students[i];
if( search === student.name ) {
message += getReport( student );
print(message);
}
}
}
Yang Zou
6,937 PointsHi Bruce, I've been looking at your code for a long time... and still don't understand why the 'else' comes before the for loop.
This is how I understand the code and I know I am wrong (!): if the searcher doesn't click 'cancel' (search === null) or type 'quit' (search.toLowerCase() === 'quit'), the sentence 'There is no student by that name' will be displayed straight away before the for loop runs.
Please can you help me understand your code... Many thanks!
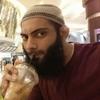
Muhammad Athar
4,004 PointsI would also recommend you to add .toLowerCase() to search in the conditional statement inside the for loop.

Bruce Kean
6,864 PointsYeah I agree. Definitely better. Thanks :)