Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial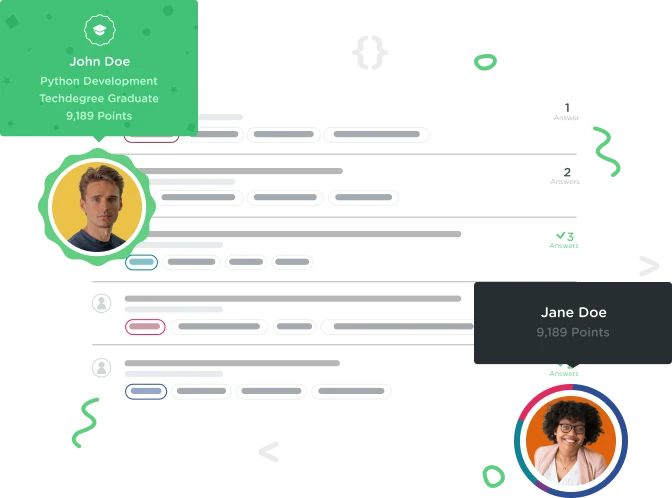
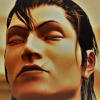
Carlton Nunes Desouza
13,447 PointsMy solution with comments
/*
Simple script to display some
math on the html page with numbers
from user input.
*/
alert("Let's do some math!");
// store prompt input as number type using +
const num1 = +prompt("Enter first number");
const num2 = +prompt("Enter second number");
// checks that variables are valid numbers, and num2 is not 0
if ( isNaN(num1) || isNaN(num2) || num2 === 0 ) {
// if not valid, alert user and do not continue block
alert("Invalid input, please reload and try again.");
// if valid continue to else statement
} else {
// create message using multiline template literals
const message = `
<h1>Math with the numbers ${num1} and ${num2}</h1>
${num1} + ${num2} = ${num1 + num2}
<br>
${num1} * ${num2} = ${num1 * num2}
<br>
${num1} / ${num2} = ${num1 / num2}
<br>
${num1} - ${num2} = ${num1 - num2}
`;
// write message to document
document.write(message);
};
notarobot
Full Stack JavaScript Techdegree Student 8,641 Pointsnotarobot
Full Stack JavaScript Techdegree Student 8,641 PointsThis is the cleanest solution i've seen to this problem. Great job!