Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial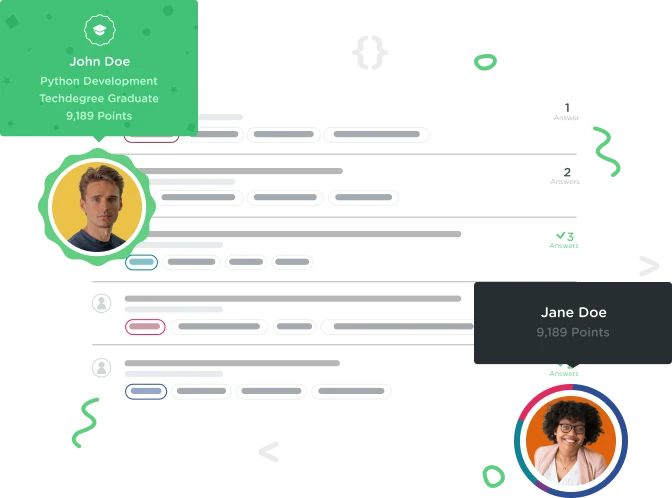
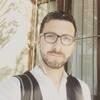
Giovanni Martínez Castagnoli
6,464 PointsMy solution with extra credit code done. Here it is using a do/while loop, what do you think?
I used an array to store the students found, after the prompt I push the results into the studentsArray, then print that array in the document, and reseting the array via var update before the prompt in the main loop.
// The Object
var students = [
{name: 'Karen',
tracks: 'Data Bases, SQL, MYSQL',
achievements: 120,
points: 8
},
{name: 'Roberto',
tracks: 'Marketing, MBA',
achievements:100,
points: 10
},
{name: 'Diego',
tracks:'PHP, SQL, .NET, JS',
achievements:120,
points:11
},
{name: 'Ignacio',
tracks:'IOS, ANDROID',
achievements:130,
points:8
},
{name: 'Giovanni',
tracks:'HTML5, CSS3, BOOTSTRAP, JS, JQ',
achievements:125,
points:9
},
{name: 'Ignacio',
tracks:'IOS, .NET',
achievements:100,
points:7
},
{name: 'Roberto',
tracks: 'Project Manager, Art Direction',
achievements:90,
points: 10
}
];
The Code
//var definition
var message = '';
var student;
var search;
// this array holds the students found
var studentsArray = [];
//print function
function print(message) {
var outputDiv = document.getElementById('output');
outputDiv.innerHTML = message;
}
//Information for Print Function
function studentList(studentsArray) {
var information = '';
for (l = 0; l < studentsArray.length; l +=1){
information += '<h2>Student: ' + studentsArray[l].name + '</h2>';
information += '<p>Tracks: ' + studentsArray[l].tracks + '</p>';
information += '<p>Points: ' + studentsArray[l].points + '</p>';
information += '<p>Achievements: ' + studentsArray[l].achivements + '</p>';
}
return information;
};
//The Loop
do {
// Update Students Arrays, empty the array for a new search.
studentsArray = [];
// ask for a student name
search = prompt('Search Student Record: type a name, for example [Dave], to end type [quit]');
// If result is null break else not found in record
if (search === null) {
break;
} else {
message ='<h2>The Student Name: ' + search + ' is not part of our Students Records</h2>';
print(message);
}
//loop through the students Array of objects and compare / search for a name
for (var i = 0; i < students.length; i += 1) {
student = students[i];
if (student.name.toLowerCase() === search.toLowerCase()){
// push every student and matching student into studentsArray
studentsArray.push(student);
//Print the result in the document
message = studentList(studentsArray);
print(message);
}
}
} while (search.toLowerCase() != 'quit')
// Go!!!
Thanks to rydavim for his help.
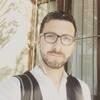
Giovanni Martínez Castagnoli
6,464 PointsMy fault :D, I already corrected it!
8 Answers
Dustin McCaffree
14,310 PointsIt looks great! Well done, friend! :) Doing those final extra credits are what I learn the most from.
Dustin
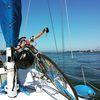
Keenan Wells
2,164 PointsAwesome post. Your comments made me understand arrays, loops, and the push method a little better I think.
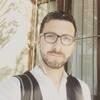
Giovanni Martínez Castagnoli
6,464 PointsThanks it took me 3 days to find the final solution, and was so simple at the end, just change a var position and empty the array, uff!
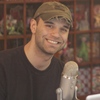
John Yzaguirre
22,025 PointsGiovanni could you explain how this one part works?
if (search === null) {
break;
} else {
message ='<h2>The Student Name: ' + search + ' is not part of our Students Records</h2>';
print(message);
}
the else is happening before the loop through student array of objects so why doesn't it always result in it saying search is not part of our student records? I know your code is right but that one part really confuses me. Great job by the way.
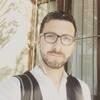
Giovanni Martínez Castagnoli
6,464 PointsHi John, let me explain, if (search === null) then break the loop, it means if you press cancel stop the loop, remember the teacher show us in the video that when you press the cancel button you get an error in the JS console, with this you prevent that error. Now if the search is not equal to anything else display message ='<h2>The Student Name: ' + search + ' is not part of our Students Records</h2>'.
That's before the for loop, so the loop only is in charge of looping through the students Array of objects and compare the results, and remember I'm using a do while loop.
Greetings
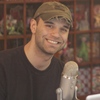
John Yzaguirre
22,025 PointsAwesome makes sense now thanks!
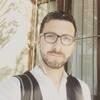
Giovanni Martínez Castagnoli
6,464 PointsIt took me 3 days! was a brain burner!!! but I did it!

Chetan Jaisinghani
2,896 PointsIf the user inputs an invalid name, does the page prompt the user again for a new name?
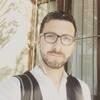
Giovanni Martínez Castagnoli
6,464 PointsChetan Jaisinghani
Yes, if you type an invalid name a message will appear in the DOM :
message ='<h2>The Student Name: ' + search + ' is not part of our Students Records</h2>'
And the Propmt will ask you again for a new name. Greetings
Eric Conklin
8,350 PointsEric Conklin
8,350 PointsI think you spelled "achievements" wrong :D