Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial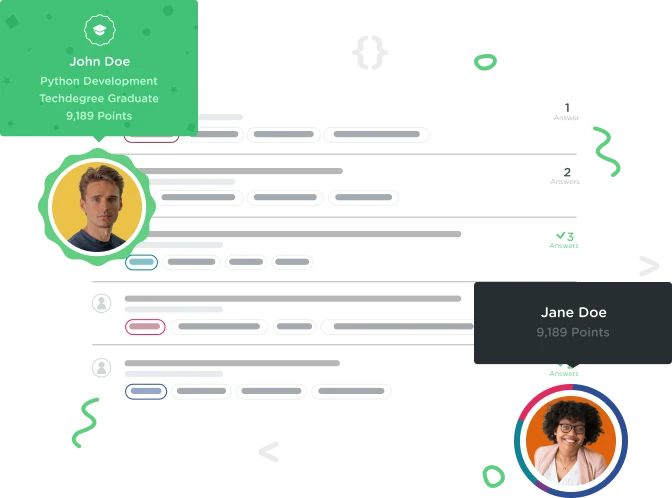

Hugo M
4,517 PointsMy solution with generic JavaScript.
Hi everyone, I thought I'd try get a deeper understanding of JavaScript and attempt this without JQuery. My solution isn't that much longer than Andrew's so I was wondering what the advantage of using the JQuery library would be in a situation where you only need a small bit of code like this? Any critiques on the code would be welcome :) Cheers.
// When user clicks on picture, open gallery.
// Launches the Gallery.
function launchGallery (image){
var imageSource = image.getAttribute("src");
var description = image.getAttribute("alt");
gallery.style.visibility = "visible";
// Attach image to gallery.
gallery.innerHTML = "<img src = '" + imageSource + "'><p>" + description + "</p><p>Click image or press escape to close.</p>";
// Gallery escape.
gallery.addEventListener('click', function (){
gallery.style.visibility = "hidden";
});
window.addEventListener('keydown', function (evt) {
if (evt.keyCode === 27){
gallery.style.visibility = "hidden";
}
});
}
// Identify Thumbnail Section
var thumbnails = document.getElementById("thumbnails");
//Create Gallery
var gallery = document.createElement("div");
thumbnails.appendChild(gallery).id = "gallery";
//Identify image links.
var galleryLinks = thumbnails.getElementsByTagName("a");
//Add event listener to each link.
for ( var i = 0; i < galleryLinks.length; i++ ) {
galleryLinks[i].addEventListener('click', function(evt){
evt.preventDefault();
launchGallery(evt.target);
});
}
1 Answer
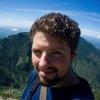
Garrett Carver
14,681 PointsI would say the advantage is that it has built in methods for each event like 'click', which just makes it simpler. I noticed in your code, it looks you put a for loop and add click event listeners on all of them to call your function. I think your code would take a higher level programmer to write and read, whereas in the jQuery code it happens in a more procedural way.
I guess the other big advantage is that jQuery it's easier to do stylish animations like fadeIn, ect. It even has methods like fadeToggle, that allow you to toggle them on and off. I like your code though, its a nice touch to add the esc key to exit the light box, I might just steal that idea from you :).