Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial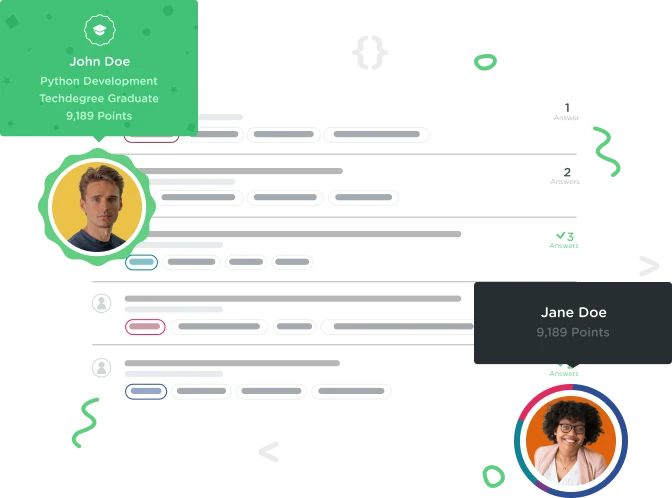
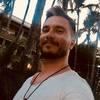
Peter Retvari
Full Stack JavaScript Techdegree Student 8,392 PointsMy solution with OpenWeathermap to get LIVE, CURRENT Weather data. 🌩😎☃❅
Hi folks, I'm happy to share my code with you. The hardest part was to set up the API with my API key, I got in a separated email because in the API documentation you only get samples, not current weather data. So if you have the email after a couple of hours of the registration process, you can use the code under Example of API call:
Example of API call
api.openweathermap.org/data/2.5/weather?q=London,uk&APPID=8af2aa7fa978da0c3dc608a85406875c
Here if you don't want to use the country, just delete it with the comma. I didn't want to waste my time to solve it to the country ID as well, so my code works only when you type node app.js and after as many cities as you want. And ofc I'm not done with the error handling part 🙈.
// Problem: get the weather data with numbers or city names by typing in the console and print out the results
// Solution: use the OpenWeather API key to get the data and display it
// Function to get the HTTPS module
const https = require('https');
// Function tp print message to the console
function printMessage(city, temp){
const message = `In ${city} the current temperature is ${temp} Celsius`;
console.log(message);
}
// Function to convert Farenheit to celsius
// Celsius = Kelvin - 273.15
function getCelsius (kelvin){
const celsius = kelvin - 273.15;
return Math.round(celsius);
}
// Connect to the OpenWeather API and get the raw data
// Example of calls: api.openweathermap.org/data/2.5/weather?q=London,uk
function getTemp (city) {
const request = https.get(`https://api.openweathermap.org/data/2.5/weather?q=${city}&APPID=8af2aa7fa978da0c3dc608a85406875c`, response => {
let body = '';
// Read the data
response.on('data', data => {
body += data.toString();
}); //data reads ends
// Parse it
response.on('end', data => {
const weather = JSON.parse(body);
printMessage(city, getCelsius(weather.main.temp));
}); // parse ends
}); //request ends
} // getTemp funciton ends
// Print out data to the console
// Make it flexible with ARGV key
const getCityTemp = process.argv.slice(2);
getCityTemp.forEach(getTemp);
Hope it will help for some student to don't mess around with the API key
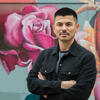
Tristan Dauvergne
7,764 PointsThanks for this, your comments really helped clarify your process!
I think it was more beneficial to not include your API key, as it forced me to look at the example API call and analyse it even closer.
1 Answer
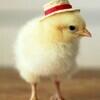
Mark Westerweel
Full Stack JavaScript Techdegree Graduate 22,378 Pointsif you add &units=metric
at the end of youe query string, you get the temperatures in Celsius. imperial
if you want Fahrenheit.
Good job on getting it to work :D
Matt Roger
15,066 PointsMatt Roger
15,066 PointsYou might not want to share your api key with everyone