Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial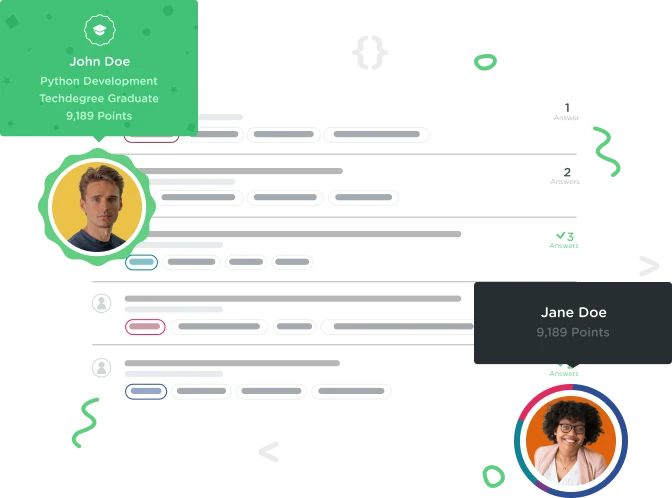
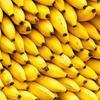
David Accomazzo
15,986 PointsMy solution works but why is this displaying "undefined" at the end?
After several hours (not even kidding) of coding I finally came up with a solution. I think I learned a lot doing this and am very satisfied with how it works except for one thing:
At the end of the quiz, I announce to the user how many questions they got right. For some reason, it's adding "Undefined" at the top of the page! Anyone know why?
The offending code should be in the quiz_ui.js file...
index.html:
<!DOCTYPE html>
<html>
<head lang="en">
<meta charset="UTF-8">
<title>Amazing Quiz</title>
<link rel="stylesheet" type="text/css" href="style.css">
</head>
<body>
<div class="grid">
<div id="quiz" class="centered grid__col--8">
<h1>Awesome Quiz</h1>
<h2 id="question" class="headline-secondary--grouped"></h2>
<p id="choice0"></p>
<button id="guess0" class="btn--default">Select Answer</button>
<p id="choice1"></p>
<button id="guess1" class="btn--default">Select Answer</button>
<footer>
<p id="progress">Question x of y</p>
</footer>
</div>
</div>
<script src="quiz.js"></script>
<script src="question.js"></script>
<script src="app.js"></script>
<script src="quiz_ui.js"></script>
</body>
</html>
quiz.js:
function Quiz() {
this.questions = [];
this.questionIndex = 0;
this.numberRight = 0;
}
Quiz.prototype.add = function(Question) {
this.questions.push(Question);
};
//Shuffle function
Quiz.prototype.shuffle = function() {
var input = this.questions;
for (var i = input.length-1; i >=0; i--) {
var randomIndex = Math.floor(Math.random()*(i+1));
var itemAtIndex = input[randomIndex];
input[randomIndex] = input[i];
input[i] = itemAtIndex;
}
return input;
}
//end Shuffle function
Quiz.prototype.next = function() {
this.questionIndex++;
}
question.js:
function Question(question, answer, fakeAnswer) {
this.question = question;
this.answer = answer;
this.fakeAnswer = fakeAnswer;
}
app.js:
var theQuiz = new Quiz();
var q1 = new Question("What is the capital of Arizona?", "Phoenix", "Tucson");
var q2 = new Question( 'According to Jerry, the husband has "first right of refusal" with regards to what bodily function of his wife?', "A sneeze", "An orgasm");
var q3 = new Question( 'Name a movie that plays in the SimTower movie theater', 'Waikiki Moon', 'Wheels on the Blunderbuss');
var q4 = new Question( 'The immortal Simpsons quote "The older they get, the cuter they ain\'t" was originally directed towards which Simpsons child?', 'Bart', 'Lisa');
var q5 = new Question( 'Which Woody Harrelson movie is referenced at some point during the movie "Robin Hood: Men In Tights"?', '"White Men Can\'t Jump"', 'Killer Instinct');
theQuiz.add(q1);
theQuiz.add(q2);
theQuiz.add(q3);
theQuiz.add(q4);
theQuiz.add(q5);
//randomize the quiz question order
theQuiz.shuffle();
var theQuestion = document.getElementById("question");
var x = Math.round(Math.random());
var a1 = document.getElementById('choice0');
var a2 = document.getElementById('choice1');
var b1 = document.getElementById('guess0');
var b2 = document.getElementById('guess1');
var score = 0;
var length = theQuiz.questions.length;
var progress = document.getElementById('progress');
var theAnswer = document.getElementsByClassName("the-answer");
var wrongAnswer = document.getElementsByClassName("wrong-answer");
function nextQuestion() {
theQuestion.innerHTML = theQuiz.questions[theQuiz.questionIndex].question;
//shuffle the answers
if ( x === 0) {
a1.innerHTML = theQuiz.questions[theQuiz.questionIndex].answer;
//add a class to the correct answer
b1.classList.remove('the-answer');
b1.classList.add('the-answer');
b2.classList.remove('the-answer');
b2.classList.add('wrong-answer');
a2.innerHTML = theQuiz.questions[theQuiz.questionIndex].fakeAnswer;
} else {
b1.classList.remove('the-answer');
a1.innerHTML = theQuiz.questions[theQuiz.questionIndex].fakeAnswer;
a2.classList.add('wrong-answer');
a2.innerHTML = theQuiz.questions[theQuiz.questionIndex].answer;
b2.classList.add('the-answer');
}
//update the progress bar
progress.innerHTML = "Question " + (theQuiz.questionIndex + 1) + " of " + length;
return true;
}
nextQuestion();
quiz_ui.js
function finishQuiz() {
var finishHTML;
finishHTML += '<h1>You Finished the Quiz!</h1>';
finishHTML += '<h2>You answered ';
finishHTML += theQuiz.numberRight;
finishHTML += ' out of ';
finishHTML += (theQuiz.questionIndex + 1);
finishHTML += ' questions correctly!';
document.getElementById('quiz').innerHTML = finishHTML;
}
theAnswer = document.getElementsByClassName("the-answer");
theAnswer[0].onclick = function (){
console.log('right');
theQuiz.numberRight++;
if (theQuiz.questionIndex < length -1) {
theQuiz.questionIndex++;
nextQuestion();
} else {
finishQuiz();
}
}
wrongAnswer = document.getElementsByClassName("wrong-answer");
wrongAnswer[0].onclick = function () {
console.log('wrong');
if (theQuiz.questionIndex < length -1) {
theQuiz.questionIndex++;
nextQuestion();
} else {
finishQuiz();
}
}
1 Answer
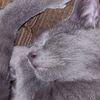
Kerriann Grossi
Courses Plus Student 10,253 Pointsvar finishHTML is undefined. I updated your code based off of what we did with song.js and that fixed it.
function finishQuiz() {
var finishHTML = '<hi>';
finishHTML += 'You Finished the Quiz!';
finishHTML += '</h1>';
Liang Lyu
2,385 PointsLiang Lyu
2,385 Pointsthe problem is that you defined a variable finishHTML but you didn't initialize it with value.