Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial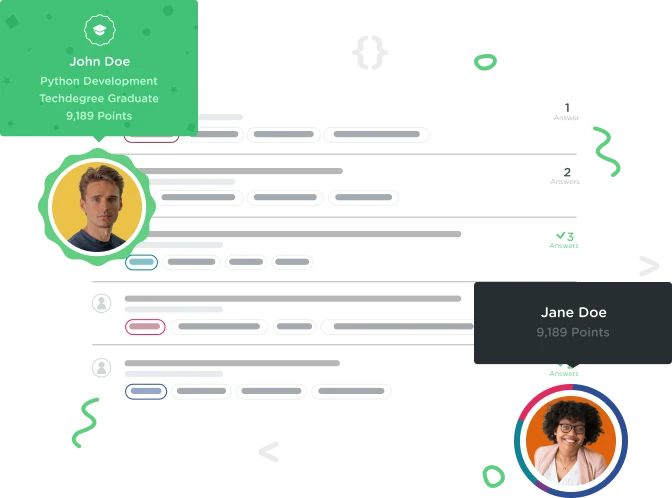
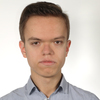
Lukas Korol
2,649 PointsMy Story Maker Challange(game). Any suggestions?
This is my Story Maker Challange. It's a game about fight between me and computer.
console.log("Start a game");
<script>alert("Welcome guest on our fighting game!");
var question = prompt("Are you ready to play this game?");
if(question === "ready")
{
var points = 25;
var name = prompt("First I would like to know what's your name?");
alert("Ok "+name+" let's create your character of warrior. \n \
You should now that you have "+points+" points to allocate.\n \
You have three features: attack, defence, strength");
var attack = prompt("Define your attack. Write a number. \n REMEMBER that \
you have "+points+" to allocate on all features!");
if(attack >= 0 && attack <= points)
{
points -= attack;
//algorithm to radomly define attack based on input value
attack = Math.floor((Math.random() * attack) + 1);
}
else
{
alert("Incorrect value of attack!");
}
var defence = prompt("Define your defence. Write a number. REMEMBER that \
you have "+points+" to allocate on all features!");
if(defence >= 0 && defence <= points)
{
points -= defence;
//algorithm to radomly define defence based on input value
defence = Math.floor((Math.random() * defence) + 1);
}
else
{
alert("Incorrect value of defence!");
}
var strength = prompt("Define your strength. Write a number. REMEMBER that \
you have "+points+" to allocate on all features!");
if(strength >= 0 && strength <= points)
{
points -= strength;
//algorithm to radomly define strength based on input value
strength = Math.floor((Math.random() * defence) + 1);
}
else
{
alert("Incorrect value of strength!");
}
//Creating a computer warrior
console.log("Creating a computer warrior");
var comp_attack = Math.floor((Math.random() * 10) + 1),
comp_defence = Math.floor((Math.random() * 10) + 1),
comp_strength = Math.floor((Math.random() * 10) + 1);
//Simply simulator of fight
var myPoints = 0,
compPoints = 0;
//compare value of attack
console.log("compare value of attack");
if(attack > comp_attack)
{
myPoints += 1;
}
else if(attack < comp_attack)
{
compPoints += 1;
}
//compare value of defence
if(defence > comp_defence)
{
myPoints += 1;
}
else if(defence < comp_defence)
{
compPoints += 1;
}
//compare value of strength
if(strength > comp_strength)
{
myPoints += 1;
}
else if(strength < comp_strength)
{
compPoints += 1;
}
//Checking who win
console.log("Checking who win");
var winner;
if(myPoints > compPoints)
{
winner = name;
}
else if(compPoints > myPoints)
{
winner = "Opponent";
}
else
{
winner = "Draw";
}
//Write result of fight
console.log("Write result of fight");
document.write("Fight finished!. Let's check your stats "+name+" and your opponent <br> \
Your stats: <br> \
Attack: "+attack+" <br> \
Defence: "+defence+" <br> \
Strength: "+strength+" <br> \
<br> \
Oponent stats:<br> \
Attack: "+comp_attack+"<br> \
Defence: "+comp_defence+"<br> \
Strength: "+comp_strength+"<br> \
<br> \
The winner is:"+winner+"<br> \
Congratulation and thank's for honest fight!");
}
else
{
document.write("Come back later!");
}
2 Answers

Will Parry
11,985 PointsHow about coming up with a list of available attacks, defenses, and strengths (e.g., for attacks - punch, kick, suplex)? Then come up with a random defense from the computer. Given the attack vs. defense, assign points accordingly. You could mirror this for defenses and strengths. It's not exactly Street Fighter but it brings a bit of strategy into your game instead of complete randomness.
Let me know if you decide to take it on!

Iain Simmons
Treehouse Moderator 32,305 PointsMy first suggestion is to remove <script>
from the third line, since it shouldn't be inside a JavaScript file!
Second, the player only gets 25 points but the computer gets 30? That doesn't seem fair... :)
Third, you should indicate to the player that they need to specifically write 'ready' to play the game. Maybe even allow just a 'y' instead.
Fourth, you're getting the attack, defence and strength as strings, so you should convert them to integers before using them. Use parseInt() for this.
Fifth, if the player puts an incorrect/invalid value to allocate to attack/defence/strength, they are alerted that it is incorrect, but the script continues anyways. Try putting each of those allocations into a function, then you can call the function again if they do it incorrectly.
In general though, great idea! Keep working on it!