Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial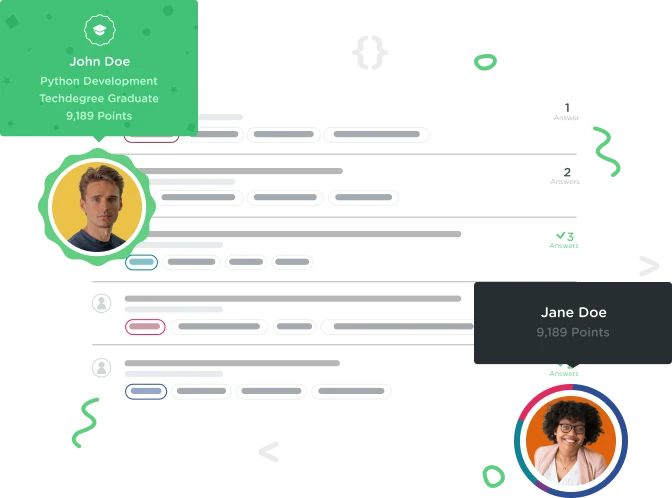

Glen Segers
1,223 Pointsmy take on the challenge, feel free to give some input
// random number between 1 and user selected
var num = prompt("pick your number"); var random = Math.floor(Math.random()*parseInt(num)) +1; alert("The random number is " + random);
// random number between 2 user selected numbers
var num = prompt("pick your lowest number"); var num2 = prompt("pick your highest number"); var random = Math.floor(Math.random()*parseInt(num2 - num)) +parseInt(num); alert("The random number is " + random);

Glen Segers
1,223 Pointsthank you both for the input, ill kepe it in mind. was my first try from scratch and uploading it
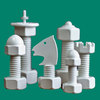
Steven Parker
230,274 PointsUse the instructions for code formatting in the Markdown Cheatsheet pop-up below the "Add an Answer" area.
Or watch this video on code formatting.
1 Answer
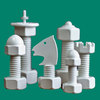
Steven Parker
230,274 PointsYou can't perform subtraction on string inputs before converting them into a number, but it may seem to work because of implicit type conversion. You'll need to convert each one individually and then you can subtract them.
parseInt(num2 - num)
parseInt(num2) - parseInt(num)
And rather than performing a conversion multiple times on the same string, you could convert it just once and assign the result to a new variable. Then use that variable as many times as needed.
Also, in both cases your random numbers may include the low limit (1 or the user input), but will not include the high limit. If you wish to include the high limit, add one to the number you multiply by.
Matthew Lanin
Full Stack JavaScript Techdegree Student 8,003 PointsMatthew Lanin
Full Stack JavaScript Techdegree Student 8,003 PointsJust fyi, if you hit the "Markdown Cheatsheet" link when making a post, it will show you how to format your code to make it easier to read on here, like this.
But your work looks good to me, running well when I try it.