Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial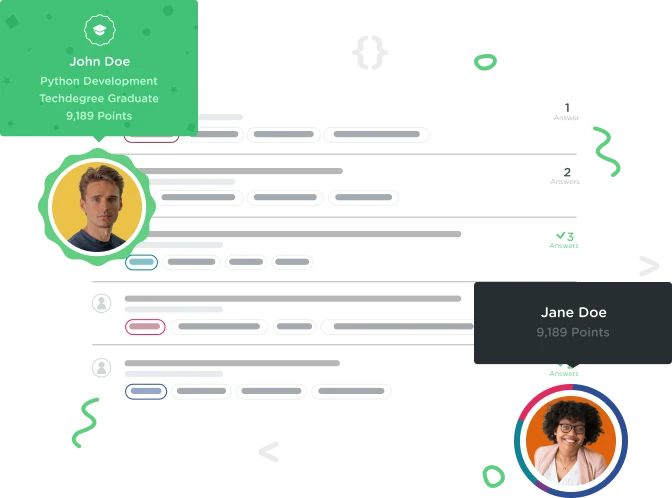

Adam Harms
9,826 Pointsmy variation on the list in python
In my code I cannot figure out why when I add a duplicate item it shows up in my "show_list" function and is printed out when completed. It is not consistent and is replaced when there is a another duplicate added in the list. Any help would be appreciated. I also have a few print statements in there to help but you can disregard those.
import os
from builtins import str
#change locations of an item
#option of removing list item by index
grocery_list = []
def clear_screen():
os.system("cls" if os.name == "nt" else "clear")
def directions():
clear_screen()
print('Input items to add to your grocery list.')
print('Input "Done" when finished with your list.')
print('Input "Remove" to remove an item from your list.')
print('Input "Show" to view your list.')
print('Input "location" to change an items location\n')
user_input()
def user_input():
# clear_screen()
grocery = input('Please input your item: ')
verify(grocery)

Adam Harms
9,826 Pointsdef remove_list():
#clear_screen()
x=1
l=0
while l < len(grocery_list):
print('{}. {}'.format(x, grocery_list[l]))
x+=1
l+=1
list_len = len(grocery_list)
remove_item = input('What item number would you like to remove? ')
try:
remove_item = abs(int(remove_item))
except ValueError:
print('\nPlease input a valid number.')
remove_list()
if remove_item > list_len or remove_item<=0:
print('\nPlease input a valid number.')
remove_list()
else:
remove_item -=1
remove = grocery_list.pop(remove_item)
print('{} have been removed.\n'.format(remove))
user_input()
def check_dup(grocery):
x=0
#if len(grocery_list) == x:
# add_to_list(grocery)
#else:
while x < len(grocery_list):
if grocery in grocery_list[x]:
print('"{}" is already in list.\n'.format(grocery))
user_input()
else:
x +=1
print('going to add list')
add_to_list(grocery)
```

Adam Harms
9,826 Pointsdef add_to_list(grocery):
# clear_screen()
grocery_list.append(grocery)
#print()
print('You have added {} to your list and it has {} items in it.'.format(grocery, len(grocery_list)))
user_input()
def show_list():
x=1
l=0
print('\nHere is your list:')
while l < len(grocery_list):
print('{}. {}'.format(x, grocery_list[l]))
x+=1
l+=1
print("\n")
def change_locations():
compted_list()
first_location = input('Please enter the item number that you want to move: ')
#final_location = input('Please enter the location you want to move it to: ')
try:
first_location = abs(int(first_location))
except ValueError:
print('Please enter a valid item number.\n')
change_locations()
if first_location > len(grocery_list) or first_location <=0:
print('Please enter a valid item number.\n')
change_locations()
else:
final_location = input('Please enter the location you want to move it to: ')
try:
final_location = abs(int(final_location))
except ValueError:
print('Please enter a valid item location.\n')
change_locations()
if final_location > len(grocery_list) or final_location <=0:
print('Please enter a valid item location.\n')
change_locations()
else:
item = grocery_list.pop(first_location-1)
grocery_list.insert(final_location-1, item)
x=1
l=0
print('\nHere is your list:')
while l < len(grocery_list):
print('{}. {}'.format(x, grocery_list[l]))
x+=1
l+=1
print("\n")
user_input()
directions()
user_input()
1 Answer

Dave Edwards
3,861 PointsHI Adam,
I am no expert but looks like a few things going on here. Firstly I have reworked you check_dup function to the following:
def check_dup(grocery):
if grocery in grocery_list: #no need to go through each item iterating by one each time
print('"{}" is already in list.\n'.format(grocery))
user_input() #get the user to re-enter another grocery
else:
print('going to add list')
grocery_list.append(grocery)
print (grocery_list)
Essentially this checks whether the grocery value is in any part of the grocery_list. If it is then user_input() is called again, if not then it will append (add to the end of the list) the grocery_list.
Your show_list function can be re-worked to get rid of the iteration as well. You can use for x in list like so:
def show_list():
x=1
print('\nHere is your list:')
for item in grocery_list:
print('\n{}. {}'.format(x, item))
x+=1

Dave Edwards
3,861 PointsTried my best but a little tricky with the code over multiple pastes. Feel free to re-post all the code in one snippet!

Dave Edwards
3,861 PointsTo be a little cleaner on the show list you can turn the index and value in to a tuple using enumerate like so:
def show_list():
x=1
print('\nHere is your list:')
#name the index value num and the grocery name as item.
#start= is what you want the starting index value to be i.e. not 0
for num, item in enumerate(grocery_list, start=1):
print('\n{}. {}'.format(num, item))
x+=1

Adam Harms
9,826 PointsThanks for the assist. I have no idea what what wrong and why it was doing what it was doing but it works great now. Thanks for the insight and the help.

Dave Edwards
3,861 PointsHope it helped!
Adam Harms
9,826 PointsAdam Harms
9,826 Points