Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial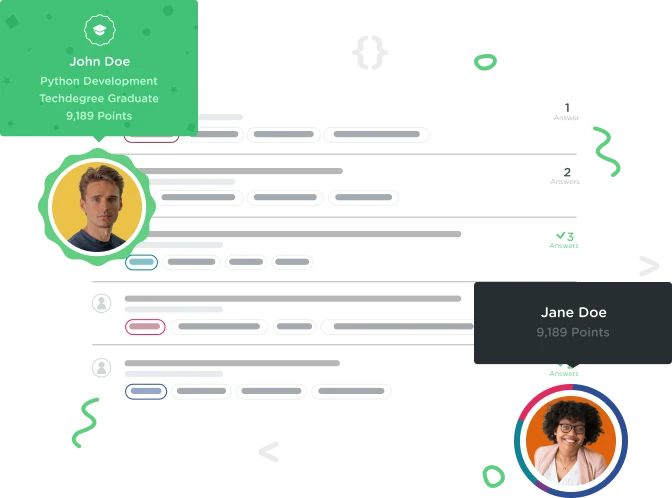
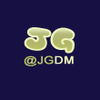
Jonathan Grieve
Treehouse Moderator 91,253 PointsMy variation on the python number game
Hi all,
Here's my variation of the Python Basics number game. What I want to do is let the user know how many attempts it took to get to the right answer.
# import random module from the standard library
import random
#generate a random number between 1 and 10
secret_num = random.randint(1,10)
attempts = []
while True:
#get an number guess from the player
guess = int(input("Guess a number between 1 and 10: "))
#compare guess to secret number
if (guess==secret_num):
print("You got it! My number was {}".format(secret_num))
break;
else:
print("Sorry, that's not the one")
for attempt in attempts:
attempt = attempt + 1
#print hit/miss
print("You did it in {} tries.".format(attempts))
First, I tried simply adding to the attempts
variable with a loop but the interpreter kept telling me int()
which I took to be the data type of attempts was not an interable.
But I know an array is an iterable so I tried an empty one.
As an example, here's what I get.
Guess a number between 1 and 10: 4
Sorry, that's not the one
Guess a number between 1 and 10: 6
Sorry, that's not the one
Guess a number between 1 and 10: 2
You got it! My number was 2
You did it in [] tries.
I feel like I'm getting close. any ideas? :)
1 Answer
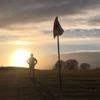
Stuart Wright
41,120 PointsHow about this? Have commented out lines of yours which I removed, and put comments on same lines as my own additions:
# import random module from the standard library
import random
#generate a random number between 1 and 10
secret_num = random.randint(1,10)
#attempts = []
attempts = 1 # A simple counter rather than a list makes more sense to me here
while True:
#get an number guess from the player
guess = int(input("Guess a number between 1 and 10: "))
#compare guess to secret number
if (guess==secret_num):
print("You got it! My number was {}".format(secret_num))
break;
else:
print("Sorry, that's not the one")
# for attempt in attempts:
# attempt = attempt + 1
attempts += 1 # increment integer counter rather than the list logic you had above
#print hit/miss
print("You did it in {} tries.".format(attempts))
Steven Parker
231,275 PointsSteven Parker
231,275 PointsI was about to suggest the same, except you may want to set an initial value of 1 instead of 0.
Stuart Wright
41,120 PointsStuart Wright
41,120 PointsOops, yes of course, thanks. Will edit my post.
Jonathan Grieve
Treehouse Moderator 91,253 PointsJonathan Grieve
Treehouse Moderator 91,253 PointsI think I was trying to be too clever by adding a loop to the code. :)
I did start by trying attempts as a simple integer... but yea... the if statement was all I really needed. Thanks. ;)
Jonathan Grieve
Treehouse Moderator 91,253 PointsJonathan Grieve
Treehouse Moderator 91,253 PointsI even had the time to add and test more functionality to the program. Since I'm proud of my work, here's what else I've done. A different message if the number was correctly guessed first attempt.
Steven Parker
231,275 PointsSteven Parker
231,275 PointsYou don't even need to use
format
when you know what the value is: