Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial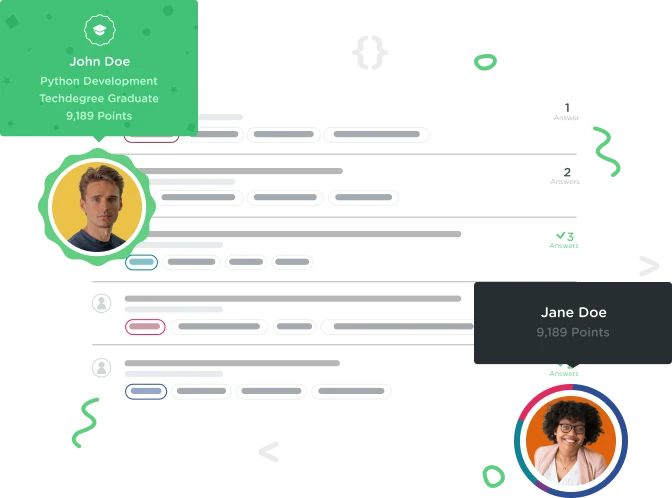

Mohammed Zeeshan
Courses Plus Student 3,302 PointsMy version did work well, but do anyone have thoughts to refactor this code further?
var html = '';
var rgbColor;
function color(){
return Math.floor(Math.random() * 256 );
}
for(i=1; i<=1000; i++) {
rgbColor = 'rgb(' + color() + ',' + color() + ',' + color() + ')';
html += '<div style="background-color:' + rgbColor + '"></div>';
}
document.write(html);
I have to use color() thrice in a statement, that looks little weird for me
3 Answers

Moira Lawrie-Martyn
8,073 PointsHere's the version I went with:
var html = '';
var colour = () => Math.floor(Math.random() * 256);
for(var i = 0; i < 16; i += 1)
{
html += '<div style="background-color:' + 'rgb(' + colour() + ',' + colour() + ',' + colour() + ')' + '"></div>';
}
document.write(html);
This was using the arrow operator as well. Down to 10 lines :)

Mohammed Zeeshan
Courses Plus Student 3,302 PointsVery clever means. Well done mate!!!

Junet Ismail
3,563 PointsChris, I was thinking along the same lines however i was unaware of the join and push functions though i did the loops the same as yours.Very clever indeed.. You could probably take it a step further. Though the code wouldn't be as clean but would cut it down by one more extra line is to inject the array join function into document.write function thus getting rid of the RGB variable entirely. As such:-
for ( j = 0; j < 10; j++ ) {
var values = [];
for ( i = 0; i < 3; i++ ) {
values.push(Math.floor(Math.random() * 256 ));
}
document.write('<div style="background-color: rgb(' + values.join(',') + ')"></div>'); }
Chris Sukovich
2,261 PointsChris Sukovich
2,261 PointsHey Mohammed,
This looks great! I did something similar because I wanted to clean up the code from the example a bit further. I iterated the random number loop 3 times, and pushed each result into an array, then joined and comma-separated the values in the array using the
.join()
method. Check out my code below: