Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial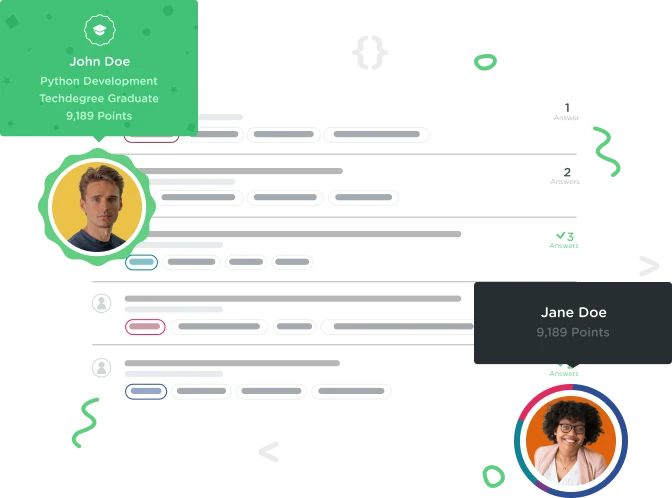
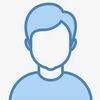
Y. Kravets
10,350 PointsMy version of Disemvoweled code for review
So guys, as usual I have tried to write it my way before actually looking at the solution. Below is what I ended up with.
The one bad thing I have already noticed about my code is that when I introduce a list of words, press enter and then continue with another list of words it only considers the last one.
The rest should work as expected. As usual comments and suggestions are greatly appreciated. I will look at the real solution now.
word_list = []
updated_word_list = []
def show_help():
print('\nThis program is designed to remove vowels from the words you provide.')
print('\nEnter DONE once you finished, \nSHOW to display the list of words you have already entered, \nHELP to display this message.')
def show_words():
counter = 1
print('\nYou currently have {} words on your list'.format(len(word_list)))
for item in word_list:
new_item = item.strip()
print('{}: {}'.format(counter,new_item))
counter += 1
show_help()
print('\nPlease enter the list of words separated by a comma now. ')
while True:
new_word = input('/>> ')
if new_word == 'DONE':
show_words()
break
elif new_word == 'SHOW':
show_words()
continue
elif new_word == 'HELP':
show_help()
continue
else:
word_list = new_word.split(',')
for item in word_list:
my_list = list(item)
while 'a' in my_list:
my_list.remove('a')
while 'e' in my_list:
my_list.remove('e')
while 'i' in my_list:
my_list.remove('i')
while 'o' in my_list:
my_list.remove('o')
while 'u' in my_list:
my_list.remove('u')
while 'y' in my_list:
my_list.remove('y')
updated_word = ''.join(my_list)
updated_word_list.append(updated_word.strip())
print('\nHere is your list with no vowels: ')
new_counter = 1
for word in updated_word_list:
print('{}: {}'.format(new_counter, word.title()))
new_counter += 1
6 Answers
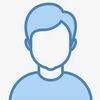
Y. Kravets
10,350 PointsBecause when I split the new_word I use a comma as an argument new_word.split(','). Essentially this means that everything before and after a comma becomes a separate element. Since we enter our words usually with spaces:
apple, orange, banana
you then get:
'apple',' orange',' banana'
If you would enter them like:
apple,orange,banana
you wouldn't have that problem, so the point of .strip() here is to ensure that if you enter it any differently you still get just a word with no extra spaces.
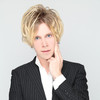
Mikael Enarsson
7,056 PointsI think that to you should use the .extend() method to append the new list items to the end of the new list, like so:
...
show_help()
continue
else:
word_list.extend(new_word.split(','))
...
That should work, I think.
Also, it would be good to move the vowel-removal functionality into a function, so that, for example, you could have an option to remove the vowels and input more words afterwards.
As far as I can see it looks really good though!
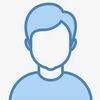
Y. Kravets
10,350 PointsThanks Mikael! I will try to implement this soon. We'll see what I will come up with. I actually think that moving the vowel-removal functionality into a function is a great idea!
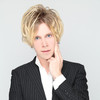
Mikael Enarsson
7,056 PointsGlad you like it ^^ I hope we'll get to see the updates XD
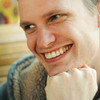
Maksym Biednov
13,459 PointsSorry, could smbdy help me to get what's the use of .strip()? I've checked documentation, but it still unclear to me. We do not use any arguments, so what are we about to remove from strings?
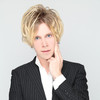
Mikael Enarsson
7,056 PointsWhen the argument is omitted or None, .strip() defaults to removing whitespaces.
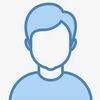
Y. Kravets
10,350 PointsJust to give an example to what Mikael said. So basically imagine you have a string: ' hello ', after applying .strip() to it you will get a string 'hello' with no spaces there anymore.
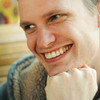
Maksym Biednov
13,459 PointsYes, I see. But why this extra spaces appear in updated_word?