Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial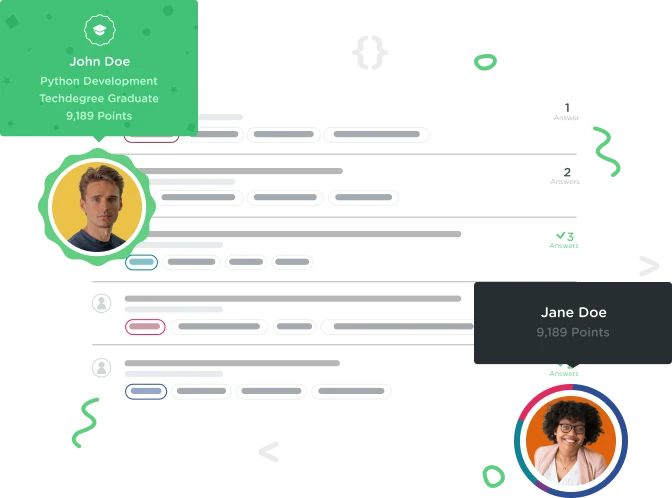

Gunhoo Yoon
5,027 PointsMy version of dungeon game needs review.
I got really obsessed to break things into pieces. Glad it made some room to extend functionality.
#Includes core game mechanic
import random, sys
import game_grid
#Returns False if nothing happens
#Else, return string according to board_presence
def game_status(pieces):
player_loc = get_last_loc('player', pieces)
monster_loc = get_last_loc('monster', pieces)
door_loc = get_last_loc('door', pieces)
if player_loc == door_loc:
return 'Player found exit!!!'
elif monster_loc == player_loc:
return 'Darkness got thicker... Monseter has swallowed you.'
else:
return False
#Retrieve last trace of game character
def get_last_loc(name, pieces):
if name in pieces:
return pieces[name]['trace'][-1] #Doing [-1:] return as list
#Move option for player, returns new co-ordinate.
def move_manual(trace, dirs, grid):
dest = None
last_loc = trace[-1]
#Keep trying until valid location or quit is fed.
while(dest not in grid):
new_dir = input('Choose direction: ').lower()
#print out set of texts based on move status.
if new_dir == 'quit':
print("\nBye\n")
sys.exit()
#Verify direction and get destination.
if new_dir in dirs:
dest = game_grid.add_vector(last_loc, dirs[new_dir])
#Attempt to go outside of wall result loss of turn.
if dest not in grid:
print("\nYou hit a wall but monster is keep looking for you.")
return last_loc
else:
#Invalid direction only generate warning
print("\nYou try to move but it appears that your anxiety has lost sense of direction." +
"You take a breath and try to move again.\n")
return dest
#Move option for monster, randomly return valid co-ordinate.
def move_random(trace, dirs, grid):
dest = None
last_loc = trace[-1]
dirs = list(dirs.values())
#Keep trying until valid co-ordinate is assigned.
while(dest not in grid):
rand_dir = random.choice(dirs)
dest = game_grid.add_vector(last_loc, rand_dir)
return dest
#From code challenge exercise.
#Takes iterable and return a list with n number of unique random choices
#Default it set to 1.
def nchoices(choices, n = 1):
result = []
#Until n choices have made
while (len(result) < n):
loc = random.choice(choices)
if loc not in result:
result.append(loc)
return result
#Assign random initial starting point to each game pieces.
def set_pieces(pieces, grid):
#Get random starting points from nchoices()
locations = nchoices(grid, len(pieces))
try:
#Take locations and assign it to each pieces trace.
for key in pieces:
piece = pieces[key]
piece['trace'].append(locations.pop())
return True
#Note: Probably be removed
except BaseException as E:
print(E)
return False
#Runs the game.
def start_game(pieces, dirs, grid):
#Basic information to print before starting the game
info = """
Contorls (quit to exit) Map
nw n ne (0, 2) (1, 2) (2, 2)
w e (0, 1) (1, 1) (2, 1)
sw s se (0, 0) (1, 0) (2, 0)
"""
#Initialize start location
set_pieces(pieces, grid)
#Examine current status <- this should always be False
status = game_status(pieces)
#Some warm up screen.
#Note: need to clear some inconsistant text formatting.
print(info)
print('All of a sudden night has fall, you hear footsteps of a monster vigorously looking for you. ' +
'You must find exit through this darkness.\n')
print("You can't see anything in this dark but you know that you were standing at {}.".format(
get_last_loc('player', pieces)))
#Run until status is either win/loss
while (status == False):
print("\nNothing has happened so far....\n")
#Play a turn.
take_turn(pieces, dirs, grid)
#Update game status.
status = game_status(pieces)
#True state of status means win/loss
if (status):
#Display whatever message returned by status
print(status)
break
#Simulate a single turn.
def take_turn(pieces, dirs, grid):
# Get trace
player_loc = pieces['player']['trace']
monster_loc = pieces['monster']['trace']
# Make move
player_move = move_manual(player_loc, dirs, grid)
monster_move = move_random(monster_loc, dirs, grid)
# Update move
player_loc.append(player_move)
monster_loc.append(monster_move)
#Includes grid related operations.
#Add two co-ordinate and return new co-ordinate as tuple.
def add_vector(vector1, vector2):
x1, y1 = vector1
x2, y2 = vector2
return (x1 + x2, y1 + y2)
#Generate grid based on (x, y) tuple co-ordinate.
def make_grid(width, height):
grid = []
for x in range(width):
for y in range(height):
grid.append((x,y))
return grid
#Runs game with game environment
import game_core, game_grid
#Used for grid generation.
width = 3
height = 3
grid = game_grid.make_grid(width, height)
#{type:{trace:[]}}
pieces = {
'player': {'trace': []},
'monster': {'trace': []},
'door': {'trace': []}
}
#{Direction: co-ordinate}
dirs = {
'n': (0, -1),
'ne': (1, -1),
'e': (1, 0),
'se': (1, 1),
's': (0, 1),
'sw': (-1, 1),
'w': (-1, 0),
'nw': (-1, -1)
}
#Start the game
game_core.start_game(pieces, dirs, grid)
1 Answer
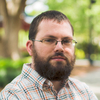
Kenneth Love
Treehouse Guest TeacherNice work!
Gunhoo Yoon
5,027 PointsGunhoo Yoon
5,027 PointsThanks Kenneth!