Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial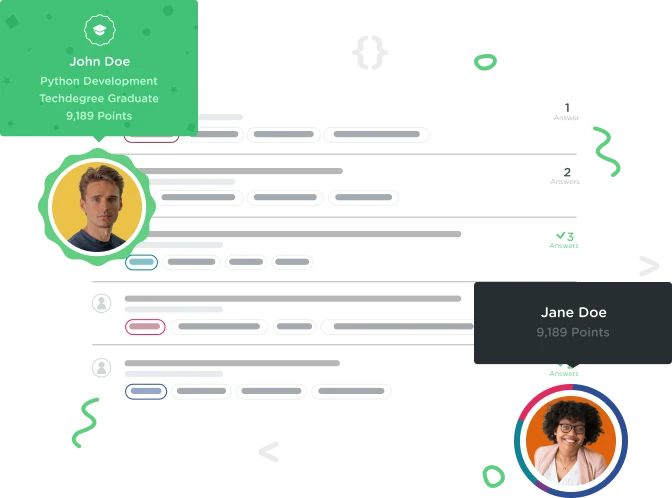
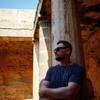
mersadajan
21,306 PointsMy version of the checkForWin method before testing
Thought of posting mine for review before testing and seeing the solution.
/**
* Method that checks if the last placed token produced a win
* @param {Array} allSpaces - Contains the two dimensional array of alls spaces of the board
* @param {Object} space - contains the space of the last played token
*/
checkForWin(allSpaces, space) {
const spaceColumn = space.x;
const spaceRow = space.y;
const player = space.owner;
const spacesBelow = allSpaces[x].slice(y+1);
const samePlayer = 0;
const amountSpacesLEFT = allSpaces.length - space.x + 1;
const amountSpacesRIGHT = space.y;
//Check for vertical win
for(let i = 0; i < spacesBelow.length; i += 1) {
if(spacesBelow[spacesBelow.length-1 - i].owner === player){
samePlayer += 1;
} else {
samePlayer = 0;
break;
}
}
//Check for diagonal win in top left and bottom right directions
for(let i = 0; i < amountSpacesLEFT;i += 1) {
if(allSpaces[space.x-1-i][space.y+1+i].owner === player){
samePlayer += 1;
} else {
break;
}
}
for(let i = 0; i < amountSpacesRIGHT;i += 1) {
if(allSpaces[space.x+1+i][space.y-1-i].owner === player){
samePlayer += 1;
} else {
break;
}
}
if (samePlayer >= 3) {
alert(`${player.name} wins!`)
} else {
samePlayer = 0;
}
//Check for diagonal win in top right and bottom left directions
for(let i = 0; i < amountSpacesLEFT;i += 1) {
if(allSpaces[space.x-1-i][space.y-1-i].owner === player){
samePlayer += 1;
} else {
break;
}
}
for(let i = 0; i < amountSpacesRIGHT;i += 1) {
if(allSpaces[space.x+1+i][space.y+1+i].owner === player){
samePlayer += 1;
} else {
break;
}
}
if (samePlayer >= 3) {
alert(`${player.name} wins!`)
} else {
samePlayer = 0;
}
//Checks for horizontal
for(let i = 0; i < amountSpacesLEFT;i += 1) {
if(allSpaces[space.x-1-i][space.y].owner === player){
samePlayer += 1;
} else {
break;
}
}
for(let i = 0; i < amountSpacesRIGHT;i += 1) {
if(allSpaces[space.x+1+i][space.y].owner === player){
samePlayer += 1;
} else {
break;
}
}
if (samePlayer >= 3) {
alert(`${player.name} wins!`)
} else {
samePlayer = 0;
}
}
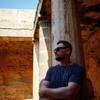
mersadajan
21,306 PointsThis was an untested method(had to fix a lot) and it was offline in visual studio code, so no workspace :) In the end I had scrap it completely. I just couldn't figure out what was wrong with it.
1 Answer
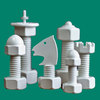
Steven Parker
230,274 PointsDebugging requires replicating the code context and running environment. Putting the project into a workspace makes it easy to share the entire environment for collaboration. The next best thing would be a REPO (like github), but that still requires the other person to have the same IDE installed.
Steven Parker
230,274 PointsSteven Parker
230,274 PointsIt's difficult for someone to evaluate a specialized method like this out of context. But you could make a snapshot of your workspace and post the link to it here.