Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial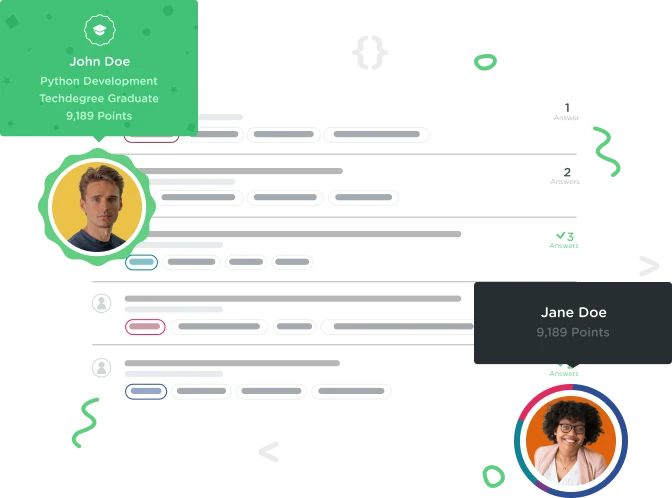
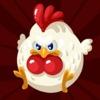
Binyamin Friedman
14,615 PointsMy Version of the Dungeon Game
I finished the game after watching the first video, so my code is pretty different:
import os
import time
import random
CELLS = [(0, 0), (1, 0), (2, 0), (3, 0), (4, 0),
(0, 1), (1, 1), (2, 1), (3, 1), (4, 1),
(0, 2), (1, 2), (2, 2), (3, 2), (4, 2),
(0, 3), (1, 3), (2, 3), (3, 3), (4, 3),
(0, 4), (1, 4), (2, 4), (3, 4), (4, 4)]
def clear_screen():
os.system('cls' if os.name == 'nt' else 'clear')
def get_locations():
locations = random.sample(CELLS, 3)
monster = locations[0];
door = locations[1];
player = locations[2];
return (monster, door, player)
def move_player(player, move):
x, y = player
if move in get_moves(player):
if move == "LEFT":
x -= 1
elif move == "RIGHT":
x += 1
elif move == "UP":
y -= 1
elif move == "DOWN":
y += 1
return x, y
def get_moves(player):
x, y = player
moves = ["LEFT", "RIGHT", "UP", "DOWN"]
if y == 0:
moves.remove("UP")
elif y == 4:
moves.remove("DOWN")
if x == 0:
moves.remove("LEFT")
elif x == 4:
moves.remove("RIGHT")
return moves
monster, door, player = get_locations()
starting_time = time.time()
while True:
clear_screen()
print("Welcome to the dungeon!")
print("You're currently in room {}".format(player))
print("\033[4m" + " |01234" + "\033[0m")
for cell in CELLS:
x, y = cell
if x == 0:
print("{}|".format(y), end = '')
if player == cell:
print('█', end = '')
else:
print('░', end = '')
if x == 4:
print('')
print("You can move {}".format(get_moves(player)))
print("Enter QUIT to quit")
move = input("> ").upper()
if move == "QUIT":
break
player = move_player(player, move)
if player == monster:
clear_screen()
print("You ran into a hungry monster! It ate you at {} :(\nGAME OVER".format(player))
break
elif player == door:
clear_screen()
print("You found the door at {} in {} seconds!\nYOU WIN".format(player, round(time.time() - starting_time, 1)))
break
1 Answer
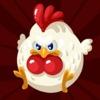
Binyamin Friedman
14,615 PointsCopy and paste it to try it out yourself!
I used some fancy looking characters to make the grid look cleaner. The weird characters on line 55 are used to underline the print statement. I also had the program track your time!