Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial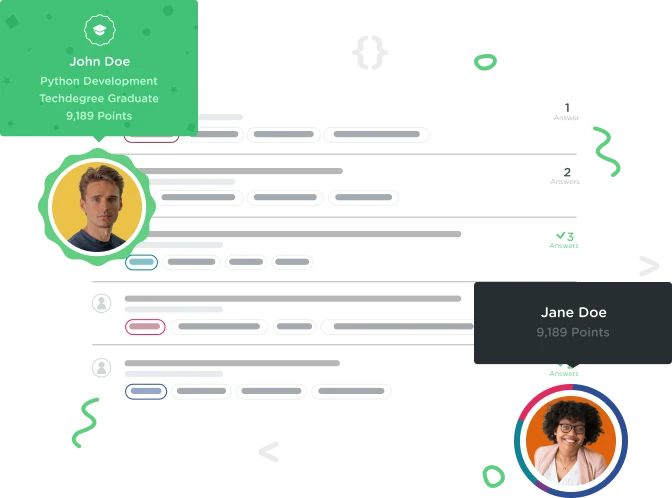

David Savard
17,081 PointsMy version of the React by Example App (with Hooks)
import React, { useState } from "react";
import GuestList from "./GuestList";
import Header from "./Header";
import Counter from "./Counter";
import ConfirmedFilter from "./ConfirmedFilter";
function App() {
const [guests, setGuests] = useState([]);
const [isFiltered, setIsFiltered] = useState(false);
const [pendingGuest, setPendingGuest] = useState("");
const[id, setId] = useState(0);
const toggleGuestPropertyAt = (property, id) => {
setGuests(prevState => prevState.map((guest) => {
if (id === guest.id) {
return {
...guest,
[property]: !guest[property]
}
}
return guest;
}))
}
const toggleConfirmationAt = id => {
toggleGuestPropertyAt("isConfirmed", id);
}
const toggleEditingAt = id => {
toggleGuestPropertyAt("isEditing", id);
}
const setNameAt = (name, id) => {
setGuests(prevState => prevState.map((guest) => {
if (id === guest.id) {
return {
...guest,
name
}
}
return guest;
}));
}
const removeGuest = (id) => {
setGuests(prevState => prevState.filter((guest) => id !== guest.id));
}
const toggleFilter = () => {
setIsFiltered(prevState => !prevState);
}
const handleNameInput = e => setPendingGuest(e.target.value);
const newGuestSubmitHandler = e => {
e.preventDefault();
if (pendingGuest) {
setGuests(prevState => [
{ name: pendingGuest, isConfirmed: false, isEditing: false, id },
...prevState
]);
}
setId(prevState => prevState + 1);
setPendingGuest("");
}
const attendingGuests = guests.filter(guest => guest.isConfirmed);
return (
<div className="App">
<Header
pendingGuest={pendingGuest}
handleNameInput={handleNameInput}
newGuestSubmit={newGuestSubmitHandler}
/>
<div className="main">
<ConfirmedFilter
toggleFilter={toggleFilter}
isFiltered={isFiltered}
/>
<Counter
total={guests.length}
attending={attendingGuests.length}
/>
<GuestList
guests={isFiltered ? attendingGuests : guests}
toggleConfirmationAt={toggleConfirmationAt}
toggleEditingAt={toggleEditingAt}
setNameAt={setNameAt}
removeGuest={removeGuest}
pendingGuest={pendingGuest}
/>
</div>
</div>
);
}
export default App;
All the imported documents are almost similar, except for some little twists, to the Guil solution. Does anybody see any loading time issues (or a bug...) with it?
On a another note, how can I make sure this post appears into the React by Example pages?
David Savard
17,081 PointsDavid Savard
17,081 PointsHow can I make sure this post appears on the React by Example page?