Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial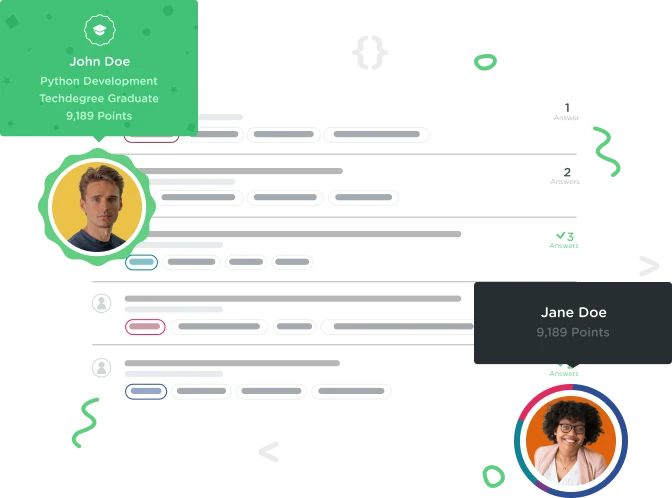
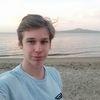
Máté Tóth-Csák
7,509 PointsMy version. What can I do better?
Heyhey. Just wanted to share my work as one possible solution for the challenge. Also would like to know how to improve, what to do better :) Thanks for any future response
function print(message) {
document.write(message);
}
var goodAnswer = 0;
var question = [
["What is the capital city of Hungary?", "budapest"],
["What is the capital city of Finnland?", "helsinki"],
["What is the capital city of New Zealand?", "auckland"]
];
while (true) {
var answer1 = prompt(question[0][0]);
if (answer1.toLowerCase() === question[0][1]) {
goodAnswer += 1;
}
var answer2 = prompt(question[1][0]);
if (answer2.toLowerCase() === question[1][1]) {
goodAnswer += 1;
}
var answer3 = prompt(question[2][0]);
if (answer3.toLowerCase() === question[2][1]) {
goodAnswer += 1;
}
break;
}
print("You answered " + goodAnswer + " questions correctly.");
1 Answer

Thomas Nilsen
14,957 PointsThere is a couple of things you can improve on;
- I would use an object to store question/answers, instead of an array.
- The while loop is unnecessary. You just break out of it anyway.
- You should loop through the questions instead if hardcoding each one. What if you had 100 questions. Would you use 100 if-statements?
With that in mind. Here is one way you could write it:
function print(message) {
document.write(message);
}
var goodAnswer = 0;
var questions = [
{ q: "What is the capital city of Hungary?", a: "budapest"},
{ q: "What is the capital city of Finnland?", a: "helsinki"},
{ q: "What is the capital city of New Zealand?", a: "auckland"}
];
questions.forEach(obj => {
var answer = prompt(obj.q.toLowerCase());
goodAnswer += (answer === obj.a) ? 1 : 0;
});
print("You answered " + goodAnswer + " questions correctly.");
Máté Tóth-Csák
7,509 PointsMáté Tóth-Csák
7,509 PointsThank you for the Answer!
Your code looks so nice and easy. Well, we did not cover the objects in the material yet (it comes next) and the task was to use arrays.
This is one of my very first codes written, thanks for the advice with the while loop. Didn't even think about it, just seems to be easier to use than for loop. :)) Will try to improve as much as possible!