Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial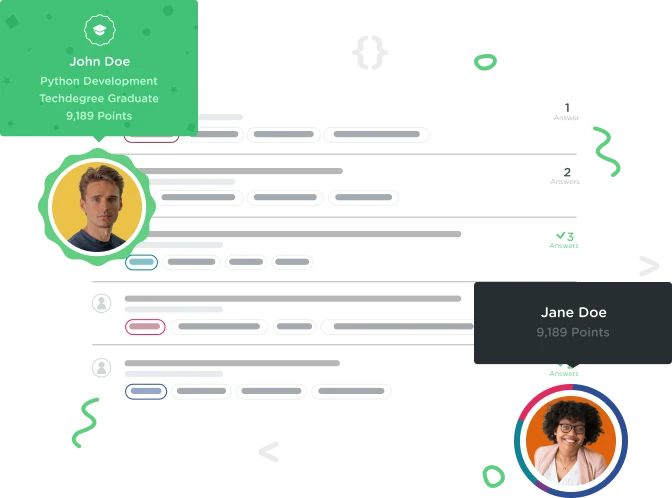

Adam Brekka
9,982 PointsMy WORKING solution to the "Disemvowel" exercise is being rejected with the "Bummer..." notification...
This code gets the job done for the challenge posed, and yet the exercise prompter is rejecting my code/calling it "incorrect"...
HUWHY???
def disemvowel(word):
#return word
vowels = ["A","E","I","O","U"]
word = word.upper()
word = list(word)
for letter in word:
try:
word.remove("A")
except ValueError:
pass
try:
word.remove("E")
except ValueError:
pass
try:
word.remove("I")
except ValueError:
pass
try:
word.remove("O")
except ValueError:
pass
try:
word.remove("U")
except ValueError:
pass
word = ''.join(word).lower()
print(word)
disemvowel("taouuoooiiieest")
7 Answers

Randy Eichelberger
1,491 PointsYou guys have a lot of code that can be taken out. Adam even goes through the effort of creating alist of vowels :p
def disemvowel(word):
vowels = ["a", "e", "i", "o", "u", "A", "E", "I", "O", "U"]
split_word = list(word)
while vowels:
try:
split_word.remove(vowels[0])
except ValueError:
del vowels[0]
word = "".join(split_word)
return word
Remember to also pay attention to DRY (Don't Repeat Yourself)
If you find yourself typing the same thing over and over again, such as the try/except, take a loot at your code and see how you can make it a little more simple. This may not seem that important at this point in your careers/education, but as you move on to larger projects you're going to want things to be as concise as possible.
And Adam your last example there had return word up at the top and down at the bottom, you want to return after all the word is complete on the word.
Basically what my code does is loop through the list created by splitting the value of word. It loops through and each time it comes across the letter in vowels[0] (so a for starters), it then removes the letter from the vowels list once it hits the ValueError exception (so when there is no longer a letter there)
After it removes the letter it goes back through and tries to find the letter again. Remember he said it's only going to look for the first instance, so we want to make sure we continue to loop through until things are right. If it finds it it removes it just like before. If it doesn't it removes itself from the vowels list. Eventually vowels will run out of things to compare and we will have our end result.
The while loop evaluates if there are any values in vowels.
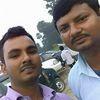
shubhamkt
11,675 PointsIn the function, you should do the following.
- Consider an empty string
- Make a list of Vowels( both lower and upper case)
- Don't change the 'word ' to list.
- Compare each letter with the array created (if x not in word) within a for loop.
- If the letter is not there, then append the empty string.
- return the word

Adam Brekka
9,982 PointsI've tried making an adjustment, and I just keep getting the notification of "Bummer! Hmm, got back letters I wasn't expecting!"
STILL NEED HELP.
def disemvowel(word):
return word
vowels = ["a","e","i","o","u","A","E","I","O","U"]
word = list(word)
for letter in word:
try:
word.remove("a")
except ValueError:
pass
try:
word.remove("e")
except ValueError:
pass
try:
word.remove("i")
except ValueError:
pass
try:
word.remove("o")
except ValueError:
pass
try:
word.remove("u")
except ValueError:
pass
try:
word.remove("A")
except ValueError:
pass
try:
word.remove("E")
except ValueError:
pass
try:
word.remove("I")
except ValueError:
pass
try:
word.remove("O")
except ValueError:
pass
try:
word.remove("U")
except ValueError:
pass
word = ''.join(word)
return word
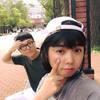
Minwook Jeong
2,453 PointsI am also having trouble with that, I've added some advises from Steven on my work, Could you look at my work?? It works well. but not works in Challenge Question
<p>
def disemvowel(word):
word = word.lower()
word = list(word)
for letter in word:
try:
word.remove("a")
except ValueError:
pass
try:
word.remove("e")
except ValueError:
pass
try:
word.remove("i")
except ValueError:
pass
try:
word.remove("o")
except ValueError:
pass
try:
word.remove("u")
except ValueError:
pass
word = "".join(word)
return word
</p>
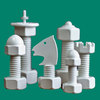
Steven Parker
231,269 PointsThe letters which are not removed should remain unchanged. But you lower-case the whole word, so any capital letters that are not vowels will not be correct in the output.
Also, I'm assuming those HTML paragraph tags are not really part of your code. The correct way to format code can be found in the Markdown Cheatsheet pop-up below the "Add an Answer" area.
Or watch this video on code formatting.

Max Phelan
1,398 PointsDecided to go the route of appending letters which aren't in the list of Vowels. Working great.
def disemvowel(word):
vowel = ['a','e','i','o','u','A','E','I','O','U']
word_list = list(word)
new_word = []
for letter in word_list:
if letter not in vowel:
new_word.append(letter)
return "".join(new_word)
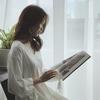
a g
Front End Web Development Techdegree Student 17,424 PointsThis is my solution to pass this challenge, I was stuck and confused about string and list, and then I found String is immutable ( because my English is not very well so maybe I pass it in the video ). So I found this data type called slide to help my problem https://stackoverflow.com/questions/4324700/python-delete-a-character-from-a-string
I hope this will help someone!
def disemvowel(word):
index =0
for letter in word:
if letter.lower() in ["a", "e", "i", "o","u"]:
word = word[:index] + word[index+1:]
else:
index +=1
return word
P.s: omg slide is the next data type we will learn @@

Jeff Mensah
5,921 PointsHere is my solution to pass the challenge. ``´ hope it helps
def disemvowel(word):
vowels = ["a", "e", "i", "o", "u", "A", "E", "I", "O", "U"]
list_word = list(word)
for vowel in vowels:
for char in word:
if vowel == char:
list_word.remove(vowel)
word = "".join(list_word).strip()
return word
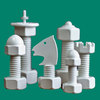
Steven Parker
231,269 Points Treehouse strongly discourages posting of solutions without explanations, and sometimes the staff or moderators will redact them.
Andrew Patella
2,270 PointsAndrew Patella
2,270 PointsVery slick, what a good idea Randy. would you mind looking at my code and telling my why I was getting rejected? I hate to admit I used your code to pass the challenge. but im still wondering why my attempt failed, any insight would be much appreciated. Thank You
when I plug it into the teamTreeHouse workspace and print the function it seems to pop out all the right letters no matter what i use for the argument. I am really stumped on it
Steven Parker
231,269 PointsSteven Parker
231,269 PointsThe challenge expects letters that are not removed to remain unchanged, but this code converts them all to lower case.
Andrew Patella
2,270 PointsAndrew Patella
2,270 PointsOhhh ok, that makes sense, Thank you
Vanessa Van Gilder
7,034 PointsVanessa Van Gilder
7,034 PointsThis is brilliant, Randy! I wish I could have thought of it on my own.
tyler bucasas
2,453 Pointstyler bucasas
2,453 Pointswhats the function of this line in your code? word = "".join(split_word)