Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial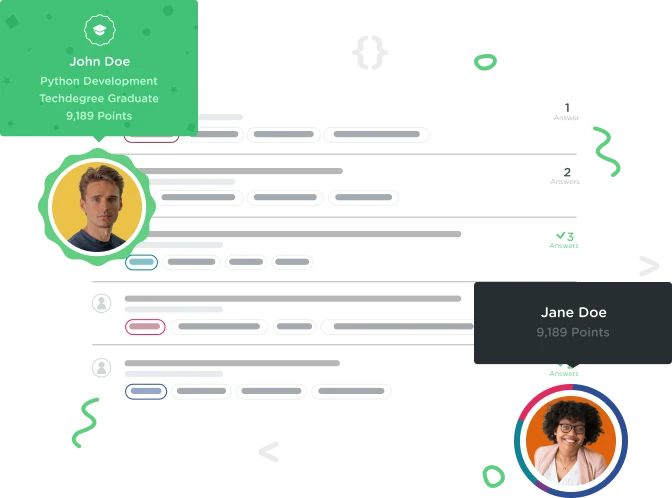

Dennis Amiel Domingo
15,357 PointsMySQL using XAMPP
To those who are using XAMPP, you can connect to the database through these steps:
- Go to the xampp > apache > conf, then open httpd.conf using your editor.
- Search for "Listen" and find the one that doesn't have a # before it. Change whatever number you have there to 3306. (i.e. Listen 3306)
- Search for "ServerName localhost" and change the number to 3306 as well. (i.e. ServerName localhost:3306)
- Save and exit.
- Now type this in your editor:
$db = new PDO("mysql:host=localhost;dbname=database;port=3306","root","");
Hope that works!
3 Answers
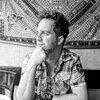
jlampstack
23,932 PointsAfter some research I found a solution that worked for me.
- Download XAMPP
- Start mySQL in XAMPP, it should be on port 3306
- In Visual Studio Code download and enable the extension "PHP Server" by baprifa (Ignore this step if you already have, or if are using a different php server. This is the one I happen to be using)
- In Visual Studio Code download and enable the extension "MySQL - MySQL management tool" by Jun Han
- Reload Visual Studio Code to ensure it's activated.
- In the Visual Studio Code Explorer you will now notice a MYSQL drop down tab at the very bottom. There should be a localhost database in there. If it's not appearing or shows an error, open XAMPP again to make sure you started mySQL
- You should now be up and running. To upload the project to phpMyAdmin in XAMPP, open the database.sql file that was downloaded from treehouse for the project. Right click anywhere on the document, and select option that says "Run MySQL Query".
- You can verify you are connected by opening the XAMPP control panel, Start the Apache Server, Open Web Browser and type localhost
- It will bring you to XAMPP page hosted locally on your computer. From there click phpMyAdmin link. On the left hand side verify that your database was uploaded.
NOTE: in this exercise the teacher is using the SQLite (PDO) driver. Instead, you will need to use the MySQL (PDO) driver.
To test that everything is working, in the inc folder, create a connection.php file. This is the code I used to test:
<?php
$db = 'mysql:host=localhost;port=3306;dbname=database';
$username = 'root';
$password = '';
$dbh = new PDO($db, $username, $password);
$query = "SELECT * FROM people";
$result = $dbh->query($query);
foreach($result as $item) {
echo "[" . $item['people_id'] . "] " . $item['fullname'] . "<BR>";
}
After saving it, right click and choose PHP Server: Serve Project to view.
NOTE: If both the Apache Server in XAMPP, and PHP Server by baprifa in VS Code are running, the PHP Server will be the one that works. To use phpMyAdmin you must stop the PHP Server.... at least that's how it is on my system.
I hope this info is helpful. I am new to this, so if there is anyone with more experience who would like to add or correct anything I may have typed, please feel free to comment.

Dennis Amiel Domingo
15,357 PointsThanks jaycode! Unfortunately, I'm not that familiar with Visual Studio Code. You may wanna try this link: https://www.youtube.com/watch?v=eE6oxEhqqoU
Hope that helps!
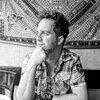
jlampstack
23,932 PointsThanks Dennis. I've seen this before. It's a good video but is for php debug. I would like to connect Visual Studio Code to phpMyAdmin (The one from Xampp) . I am not sure if this is possible.

Dennis Amiel Domingo
15,357 PointsI'll try to research more about it. :)
jlampstack
23,932 Pointsjlampstack
23,932 PointsDennis Amiel Domingo Great tip! Do you know if there is a way we can access Xampp directly through the code editor? I have Xampp and Visual Studio Code but would like to run everything from VSC if possible?