Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial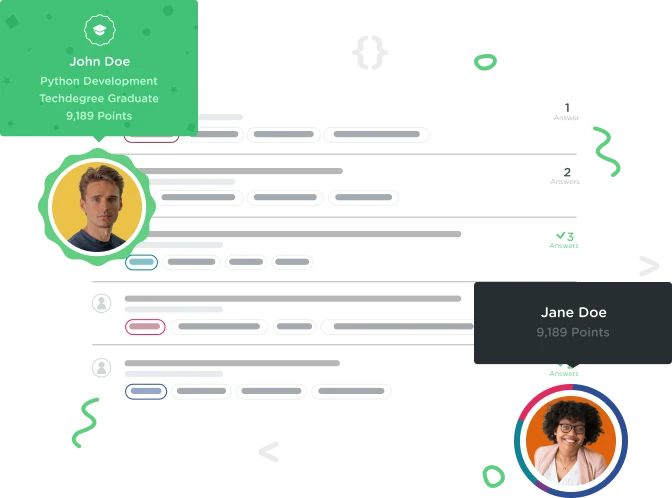

Nate Hawk
13,229 Points"\(n)" quotations don't close
It looks like the quotations aren't closing for some reason and so the rest of my code isn't registering. it all shows up green.
func fizzBuzz(n: Int) -> String {
// Enter your code between the two comment markers
for n in 1...100 {
if (n % 3 == 0) {
return "Fizz"
} else if (n % 5 == 0) {
return "Buzz"
} else if (n % 3 == 0) && (n & 5 == 0) {
return "FizzBuzz"
} else { return "\(n)"}
// End code
}}
1 Answer

Anjali Pasupathy
28,883 PointsThat's just because the quiz compiler doesn't see the string interpolation of n for what it is. The code looks green, but it functions normally. Just ignore the green-ness.
However, there are other reasons why your code is failing.
Firstly, the challenge doesn't ask you to do fizzbuzz for all the numbers from 1 to 100; it just wants you to do fizzbuzz for the parameter passed into the function (n). Because of that, you don't need the for loop. You should get rid of that.
Another thing you need to take care of is the order of your if statements. Once the condition of an if statement evaluates to true, the code block inside that statement runs and the code blocks inside all subsequent else if and else statements are ignored. This means that once you pass in an n that's divisible by both 3 and 5 (for example, 30), it'll check the first if statement, see that the number is divisible by 3, and execute just that block of code. It won't check either of the next two statements. To fix this, you should move the if statement checking for divisibility by both 3 and 5 to the top. If you do this, then all numbers divisible by both 3 and 5 will return "FizzBuzz", and any numbers that are divisible by 3 or 5, but not both, will go on to the next if statements.
The last thing you need to do is correct a typo: in the condition where you check for divisibility by both 3 and 5, you've written "n & 5 == 0". You need to change that to "n % 5 == 0".
func fizzBuzz(n: Int) -> String {
// Enter your code between the two comment markers
for n in 1...100 { // GET RID OF THIS AND ITS CORRESPONDING CLOSING BRACKET
if (n % 3 == 0) {
return "Fizz"
} else if (n % 5 == 0) {
return "Buzz"
} else if (n % 3 == 0) && (n & 5 == 0) { // MOVE THIS STATEMENT TO THE TOP
// CHANGE n & 5 TO n % 5
return "FizzBuzz"
} else { return "\(n)"}
// End code
}}
I hope this helps!