Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial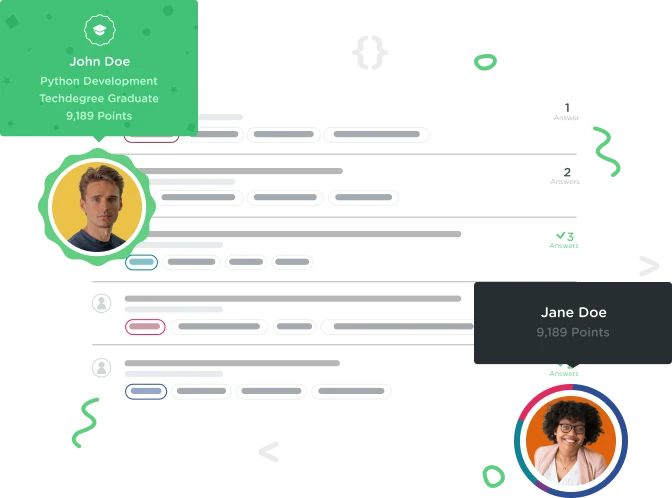

Chris Ochsenreither
4,350 Pointsname 'current_score' is not defined
When I run my code in the Python interpreter after importing Game, it says that 'current_score' is not defined.
from game import Game mygame = Game() mygame.score() Enter a player '1' or '2': 1 NameError: name 'current_score' is not defined
I thought passing self to score would make self.current_score available
class Game:
def __init__(self):
self.current_score = [0, 0]
def score(self):
pnum = input("Enter a player '1' or '2': ")
if pnum in '12':
if pnum == '1':
self.current_score[0] += 1
else:
self.current_score[1] += 1
else:
print("Invalid number")
3 Answers

Gavin Ralston
28,770 PointsIf the challenge is asking for a player argument, it's asking you to pass a representation of a player into the function. In this case the number 1 or 2, and then do something based on the value (player number) that was passed in.
So it should start something like:
def score(self, pnum):
if something:
do something
Remember to pass in self first in order to access self's attributes, and don't get caught up with the input and print statements unless you were just using that for testing out your code on your own machine. It literally wants you to take a number and update the index in the player scores just like you did in your original code. Not to take input from a user or anything.
That's also not the only way to do it. You could perform the update in a single line that isn't too terribly complicated looking and skip the if statement altogether.
Good luck! (I'm out the door for a while)
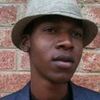
MUZ140734 Tinashe Chibwe
16,960 Pointsprecise answer
class Game: def init(self): self.current_score = [0, 0]
def score(self, player): self.current_score[1] += 1
return player

Gavin Ralston
28,770 PointsThis code doesn't throw any errors for me if I run it in the interpreter.
Is there any chance you've edited in a text editor, but haven't yet saved those changes? Like, you're reading the completed code, but you're running a version that isn't quite complete?

Chris Ochsenreither
4,350 PointsThanks! Any suggestions on how to make my code pass?

Chris Ochsenreither
4,350 PointsThat must be it! I copied and pasted the code from my text editor into IDLE and it ran. I verified the score changed with a call to mygame.current_score
Unfortunately, the Treehouse parser doesn't like my answer - "Bummer! Try again!" so I must have left out something it was looking for.

Gavin Ralston
28,770 PointsAny chance you could paste the challenge text here? I'm not sure what part of the challenge you're on.

Chris Ochsenreither
4,350 PointsAbsolutely... and thanks for taking the time to help. The challenge is:
Add a score method to Game that takes a player argument that'll be either 1 or 2. Increase that player's value in self.current_score by 1. You'll need to adjust the index (i.e. player = 1 means self.current_score[0] needs to increase).
and the code carried over from the first step is as follows:
class Game: \n def init(self): \n self.current_score = [0, 0]
(the first three lines)
Chris Ochsenreither
4,350 PointsChris Ochsenreither
4,350 PointsThanks, after trying some variations on the answer it doesn't like it when you print a notification of an exception.