Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial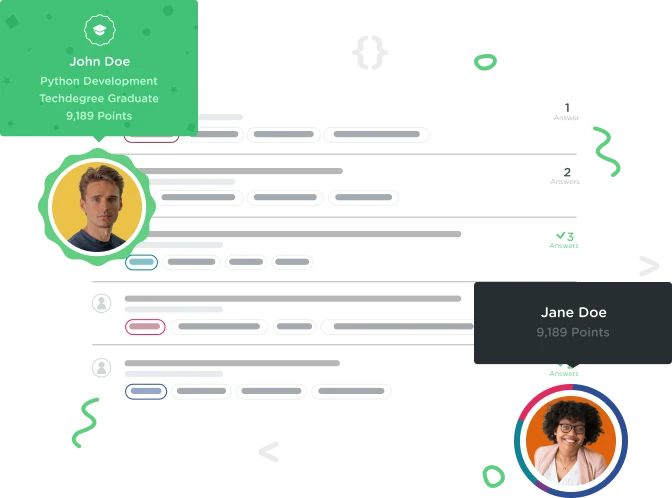
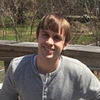
Matthew Russo
3,871 PointsName Search Not Working
Each search in the prompt box results in the message from my else statement, There is no student named ${search}.
even when it should be a match. This tells me there must be something wrong with the if statement in my if else conditional. Can anybody see what I've done wrong? My code is below:
//students.js
var students = [
{
name: 'Asma',
track: 'Front End Development',
achievements: 120,
points: 1200
},
{
name: 'Brian',
track: 'iOS Development with Swift',
achievements: 210,
points: 2150
},
{
name: 'Charlie',
track: 'PHP Development',
achievements: 170,
points: 2380
},
{
name: 'Dave',
track: 'WordPress',
achievements: 215,
points: 2190
},
{
name: 'Eve',
track: 'Rails Development',
achievements: 150,
points: 1550
}
];
//student_report.js
var message = '';
var student; //holds a student object each time the loop runs
var search;
function print(message){
var outputDiv = document.getElementById('output');
outputDiv.innerHTML = message;
}
function getStudentReport(student){
var report = `<h2>Student: ${student.name}</h2>`;
report += `<p>Track: ${student.track}</p>`;
report += `<p>Achievements: ${student.achievements}</p>`;
report += `<p>Points: ${student.points}</p>`;
return report;
}
while(true){
search = prompt('Search for a student in our directory. Type quit to exit.');
if (search === null || search.toLowerCase() === 'quit'){
break;
}
for (var i=0; i < students.length; i++){
student = students[i];
if(student.name === search){
message = getStudentReport(student);
print(message);
} else{
print(`There is no student named ${search}.`);
}
}
}
Moderator edited: Markdown added so that code renders properly in the forums. -jn
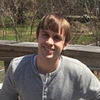
Matthew Russo
3,871 PointsHi Shay,
I added search = search.toLowerCase(); but the same problem persists.
1 Answer
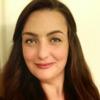
Jennifer Nordell
Treehouse TeacherHi there! It actually does work, but not in the way you're intending. It will show a result, but only in one case. You must search for "Eve" and then type "quit" for the search to display anything.
But why is that? Well, let's take a look at your for
loop. Let's assume that we're searching for "Brian". We start the for
loop and ask if it's null or quit. It isn't so we continue. Now if the search term is equal to the first student, we print that student's information, but if it isn't, we print that no results can be found for the student.
So on the first iteration, the student's name is equal to "Asma" and our search term is "Brian". We take the entirety of the outputDiv
and then replace it with "There is no student named Brian". So far, so good.
On the second iteration, the student's name is equal to "Brian" and our search term is "Brian". They match up and so we add all that student's information to the outputDiv
. So far, so good. But remember, nothing will be displayed until our loop completes.
It's the next iteration where it gets interesting. On the next iteration, the student's name will be "Charlie" and our search term is still "Brian". These do not match, so all of the information for the search result from the previous iteration is replaced with "There is no student named Brian", which is obviously not what you're intending here.
I hope maybe this helps you come up with a solution and it is possible! You're doing great!

brendon hanson
6,191 PointsGreat answer! Correct me if I'm wrong but would the solution be to add a "break" statement after the print(message)?
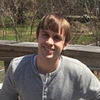
Matthew Russo
3,871 PointsThanks Jennifer! That cleared the issue up for me and the program works now. I added a break statement after print(message) as Brendan suggested in the other comment and that was part of the solution.
I also had to add the .toLowerCase() method to search AND student.name for the comparison to work. Here's what my while loop looks like now:
while(true){
search = prompt('Search for a student in our directory. Type quit to exit.');
search = search.toLowerCase();
if (search === null || search === 'quit'){
break;
}
for (var i=0; i < students.length; i++){
student = students[i];
if(student.name.toLowerCase() === search){
message = getStudentReport(student);
print(message);
break;
} else{
message += There is no student named ${search}.
;
print(message);
}
}
}
Shay Paustovsky
969 PointsShay Paustovsky
969 PointsHi Matthew,
Check for any comparison problems because : 'Eve' || 'Charlie' do not equal to 'eve' || 'charlie'.