Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial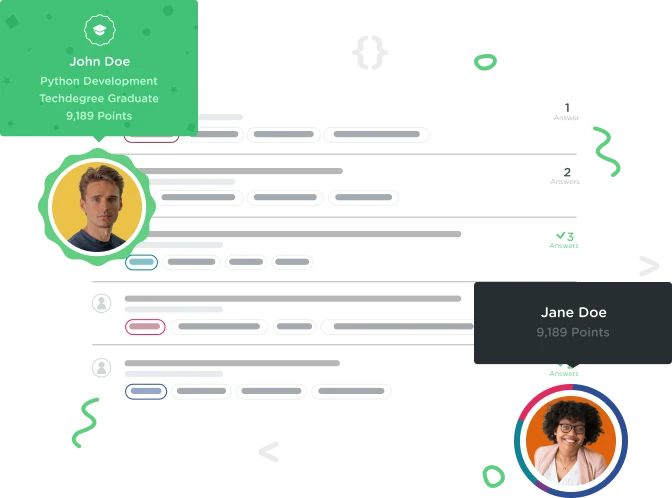

Corey Buckley
1,257 PointsNameError in DungeonGame.py
This is my attempt at completing the project with some extra things added in (e.g moving the monster, having more than one monster) and it works perfect 80% of the time only im getting this NameError every now and again. PS This has nothing to do with the challenge for which this was posted under, I couldnt figure out how to get my code to format correctly without posting under a challenge.
OUTPUT:
Welcome To The Dungeon!
☐☐☐☩☐ ☐☐☐☐☐ ☠☐☒☐☐ ☐☐☐☐☐ ☐☐☐☠☐
Try to make it to the exit before the monsters finds you! Your position is marked by a ☩ The position of the exit is marked by a ☒ The position of the monsters is marked by a ☠ Neither you or the monsters can move diagonally
You're currently in room (3, 0) Possible Moves: LEFT RIGHT DOWN
DOWN Traceback (most recent call last): File "C:\Users\Corey\Desktop\PyPro\TreehouseProject3.py", line 154, in <module> move_monster1() File "C:\Users\Corey\Desktop\PyPro\TreehouseProject3.py", line 111, in move_monster1 new_index = monster1_current_index + monster1_move NameError: name 'monster1_move' is not defined
import random
CELLS = [(0,0), (1,0), (2,0), (3,0), (4,0),
(0,1), (1,1), (2,1), (3,1), (4,1),
(0,2), (1,2), (2,2), (3,2), (4,2),
(0,3), (1,3), (2,3), (3,3), (4,3),
(0,4), (1,4), (2,4), (3,4), (4,4),]
cells = CELLS.copy()
grid = ["☐", "☐", "☐", "☐", "☐",
"☐", "☐", "☐", "☐", "☐",
"☐", "☐", "☐", "☐", "☐",
"☐", "☐", "☐", "☐", "☐",
"☐", "☐", "☐", "☐", "☐"]
def get_locations(insert_CELLS_when_called, insert_cells_when_called):
global monster1_position, monster2_position, door_position, player_position
monster1_position = random.choice(CELLS)
cells.remove(monster1_position)
monster2_position = random.choice(CELLS)
cells.remove(monster2_position)
door_position = random.choice(cells)
cells.remove(door_position)
player_position = random.choice(cells)
return monster1_position, monster2_position, door_position, player_position
def print_grid():
grid = ["☐", "☐", "☐", "☐", "☐",
"☐", "☐", "☐", "☐", "☐",
"☐", "☐", "☐", "☐", "☐",
"☐", "☐", "☐", "☐", "☐",
"☐", "☐", "☐", "☐", "☐"]
players_current_index = CELLS.index(player_position)
grid[players_current_index] = "☩"
doors_index = CELLS.index(door_position)
grid[doors_index] = "☒"
monster1_current_index = CELLS.index(monster1_position)
grid[monster1_current_index] = "☠"
monster2_current_index = CELLS.index(monster2_position)
grid[monster2_current_index] = "☠"
print("".join(grid[0:5]))
print("".join(grid[5:10]))
print("".join(grid[10:15]))
print("".join(grid[15:20]))
print("".join(grid[20:25]))
def get_moves():
global player_position, move
move = ["LEFT", "RIGHT", "UP", "DOWN"]
if player_position == (0,0):
move.remove("LEFT")
move.remove("UP")
elif player_position == (4,0):
move.remove("RIGHT")
move.remove("UP")
elif player_position == (0,4):
move.remove("LEFT")
move.remove("DOWN")
elif player_position == (4,4):
move.remove("RIGHT")
move.remove("DOWN")
elif player_position[0] == 0:
move.remove("LEFT")
elif player_position[0] == 4:
move.remove("RIGHT")
elif player_position[1] == 0:
move.remove("UP")
elif player_position[1] == 4:
move.remove("DOWN")
global possible_moves
possible_moves = move
return possible_moves
def move_player(move):
global player_position
current_index = CELLS.index(player_position)
if move == "UP":
new_index = current_index - 5
elif move == "DOWN":
new_index = current_index + 5
elif move == "LEFT":
new_index = current_index - 1
elif move == "RIGHT":
new_index = current_index + 1
player_position = CELLS[new_index]
return player_position
def move_monster1():
global monster1_position
global monster1_move
monster1_current_index = CELLS.index(monster1_position)
if monster1_position[0] == 0:
if monster1_position == (0,0):
monster1_move = random.choice([1, 5])
elif monster1_position == (0,4):
monster1_move = random.choice([1, -5])
else:
monster1_move = random.choice([1, 5, -5])
elif monster1_position[0] == 4:
if monster1_position == (4,0):
monster1_move = random.choice([-1, 5])
elif monster1_position == (4,4):
monster1_move = random.choice([-1, -5])
else:
monster1_move = random.choice([-1, 5, -5])
new_index = monster1_current_index + monster1_move
monster1_position = CELLS[new_index]
return monster1_position
def move_monster2():
global monster2_position
global monster2_move
monster2_current_index = CELLS.index(monster2_position)
if monster2_position[0] == 0:
if monster2_position == (0,0):
monster2_move = random.choice([1, 5])
elif monster2_position == (0,4):
monster2_move = random.choice([1, -5])
else:
monster2_move = random.choice([1, 5, -5])
elif monster2_position[0] == 4:
if monster2_position == (4,0):
monster2_move = random.choice([-1, 5])
elif monster2_position == (4,4):
monster2_move = random.choice([-1, -5])
else:
monster2_move = random.choice([-1, 5, -5])
new_index = monster2_current_index + monster2_move
monster2_position = CELLS[new_index]
return monster2_position
get_locations(CELLS, cells)
get_moves()
print("\nWelcome To The Dungeon!\n")
print_grid()
print("\nTry to make it to the exit before the monsters finds you!")
print("Your position is marked by a ☩")
print("The position of the exit is marked by a ☒")
print("The position of the monsters is marked by a ☠")
print("Neither you or the monsters can move diagonally")
print("\nYou're currently in room {}".format(player_position))
print("Possible Moves: {}".format(" ".join(possible_moves)))
while True:
move = input("> ")
if move in possible_moves:
move_player(move)
move_monster1()
move_monster2()
print()
print_grid()
if player_position == monster1_position:
print("\nYou entered a room containing a monster...You lose!")
break
if player_position == door_position:
print("\nYou made it to the exit. You win!")
break
get_moves()
print("\nCurrent Position: {}".format(player_position))
print("Possible Moves: {}".format(" ".join(possible_moves)))
else:
print("\nERROR! Please ensure your input is uppercase and that your move is possible.\n")
print_grid()
print("Current Position: {}".format(player_position))
print("Possible Moves: {}".format(" ".join(possible_moves)))
1 Answer
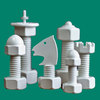
Steven Parker
231,269 PointsIn the "move_monster1 " function, the definition of "monster1_move" depends on "monster1_position[0] == 0
" or "monster1_position[0] == 4
". If it has any other value, then "monster1_move" will remain undefined.
There's a similar issue where the same logic is used in the "move_monster2" function.
It looks like more code may be needed to handle all possible cases properly.
Corey Buckley
1,257 PointsCorey Buckley
1,257 PointsTook some time but got it fixed. Didn't account for monsters not being able to move up in top part of grid or down in bottom part of grid and I had to restructure if else elif part. Thanks so much! ?