Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial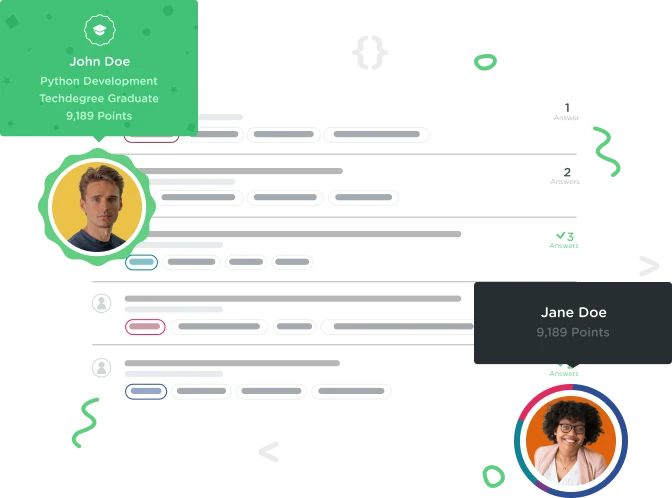

Chris Feltus
3,970 PointsNaming Jquery Functions
I have been working with anonymous functions up until now, and would like to use some named functions. Below is an anonymous function and it works just fine
The code for a named function below appears to work, but I just wanted to verify I am doing this correctly.
$('button').click(function doSomething() {
$(this).prev().show();
$(this).remove();
});
Thanks
3 Answers

Tyler Gillies
334 PointsIt is less typing to just do
$('button').click(function(){
$(this).prev().show();
$(this).remove();
});
The idiomatic way to use named functions is to do:
function doSomething(thing){
return thing + 1
}
doSomething(3);
A lot of times in javascript you never use the name of the function because it's in a callback. (Like your example), you only need to name a function if you're going to explicitly call it by name.
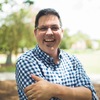
Dave McFarland
Treehouse TeacherYou can also write it this way:
function doSomething() {
$(this).prev().show();
$(this).remove();
};
$('button').click(doSomething);
The named function goes outside of the event handler. In addition, you pass just the name of the function -- doSomething
-- to the .click()
method. Don't add parentheses to doSomething in the click method. This, for example is wrong: $('button').click(doSomething());
However passing anonymous functions is the more common way to do it.

shezazr
8,275 Pointsit make sense to used named function if you use some functionality in different places..