Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial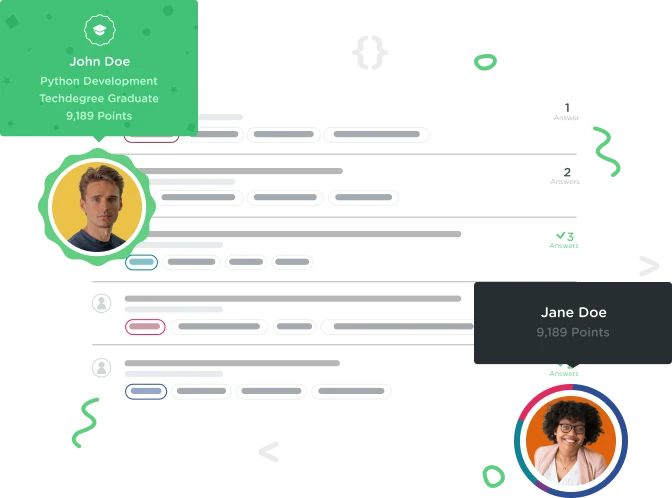
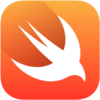
Antonija Pek
5,819 PointsNaming parameters
So by default second, third etc. are external names, even if it's our custom function, and we see them when providing an arguments and this is something Apple decided is, I guess, user friendly?
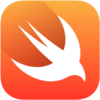
Antonija Pek
5,819 PointsWe had example in video, and it goes like this:
func calculateArea(length: Int, width: Int) -> Int {
return length * width
}
... And when we are calling function we have this:
calculateArea(12, width: 43)
So width parameter name is visible while lenght isn't.
3 Answers
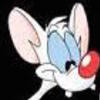
Jake Adams
1,608 PointsApple encourages a specific naming convention for functions. Typically, the function name itself will end with the first variable in the argument list.
func calculateAreaWithLength(length: Double, width: Double) -> Double {}
calculateAreaWithLength(10.4, width: 5)
If you want to give the first argument and external name, you can use the _
character.
func calculateArea(length _: Double, width: Double) -> Double {}
calculateArea(length: 10.4, width: 5)
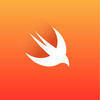
Steven Deutsch
21,046 PointsHey Antonija Pek,
So your question is regarding naming parameters in Swift.
Function parameters have both an external name and a local name.
You define the external parameter name before the local parameter name, separated with a space. The external parameter name is used to label the arguments that you pass into a function when you call it. Whereas, the local parameter name is used inside of the function itself.
func anyGivenFunction(externalName localName: String) -> String {
// local parameter names are used here
}
anyGivenFunction(externalParameter) // external parameter names are used here
If no external parameter name is specified, Swift will use the parameter's local name as it's external name. When calling the function, the external name of the first parameter is omitted by default. However, if you specify an external parameter name, it is mandatory that you use that name when calling the function.
Finally, if you don't want to use an external name when you call your function, you can use an underscore _ as the external parameter name to omit it.
func anyGivenFunction(localName: String, _ anotherLocal: String) -> String {
return localName + anotherLocal
}
anyGivenFunction("No external by default", "No external name here either")
Hope this helps!
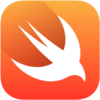
Antonija Pek
5,819 PointsThanks!
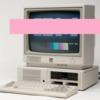
kols
27,007 PointsThis answer is correct w/ the exception that you wouldn't want to do this for the first - or, in this case, only - parameter name. (The first parameter name has no external name by default, only a local name.)
More here: Apple Docs, Swift 2.2 Functions
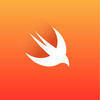
Steven Deutsch
21,046 PointsAlright, I updated my answer :P Lol

Bencheng Zheng
947 Pointsyou guys are awesome
Jake Adams
1,608 PointsJake Adams
1,608 PointsCan you provide a code example?