Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial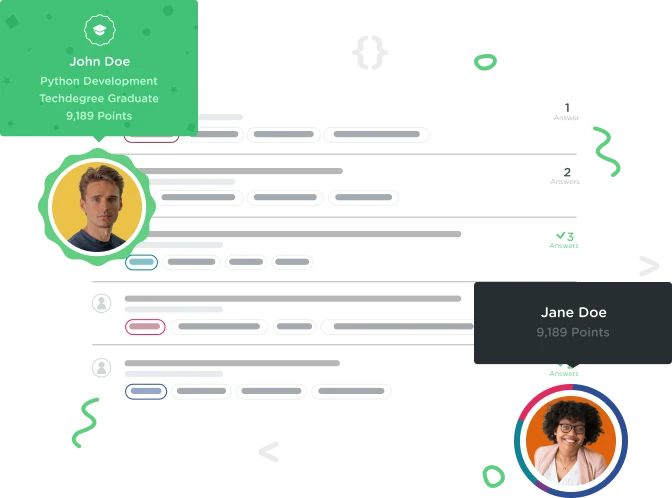

Unsubscribed User
1,653 PointsNaN Error
Hi, I have a game where the main camera follows the constantly moving character trying to dodge obstacles. It is a long platform in the sky with the player and obstacles on that platform and if you fall off you die. I get a NaN error occasionally while playing my game usually when I ma by the edges of the platform about to fall off etc. I will share the console error given and the code the problem apparently lies in. Please help me fix/change my code to get rid of the NaN error (More info: The offset was set to X:0 Y:2 Z:-5):
Console: transform.position assign attempt for 'Main Camera' is not valid. Input position is {NaN, NaN, NaN}, UnityEngine.Transform:set_position(Vector3)
Invalid AABB a
Invalid AABB a
Invalid AABB a
Assertion failed on expression: 'IsFinite(d)'
Assertion failed on expression: 'IsFinite(outDistanceForSort)'
Assertion failed on expression: 'IsFinite(outDistanceAlongView)'
(and the more Invaild Lines)
The code:
using System.Collections; using System.Collections.Generic; using UnityEngine;
public class FollowPlayer : MonoBehaviour {
public Transform player;
public Vector3 offset;
// Use this for initialization
void Start () {
}
// Update is called once per frame
void Update () {
transform.position = player.position + offset;
}
}
1 Answer
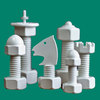
Steven Parker
231,169 PointsIt's probably a result of adding something that is not a valid value.
A result of NaN is often caused by performing math on something other than a valid numeric value. I see in your update function where you add player.position and offset, but none of the code shown indicates how either of those gets set. I might make a wild guess that offset was not set before the first update and still contained null, causing the addition operation to return NaN.
You might want to set a breakpoint at this code position and examine the values being added. If my guess is right, you'll then need to look for somewhere in other code where one (or both) of them is not being set to a valid value.
Unsubscribed User
1,653 PointsUnsubscribed User
1,653 PointsSo there is no specific code I can add to fix it?
Unsubscribed User
1,653 PointsUnsubscribed User
1,653 PointsHere is another script that may be related to the problem (Player Movement):
using UnityEngine;
public class PlayerMovement : MonoBehaviour {
void FixedUpdate() { rb.AddForce(0,0, forwardForce * Time.fixedDeltaTime);
}
Steven Parker
231,169 PointsSteven Parker
231,169 PointsIt doesn't appear to be in that module either. This is something that really requires the whole project to be loaded and make use of the debugger.
Also, when posting code, use the instructions for code formatting in the Markdown Cheatsheet pop-up below the "Add an Answer" area.
Unsubscribed User
1,653 PointsUnsubscribed User
1,653 PointsHow would one go about debugging for NaN?
Steven Parker
231,169 PointsSteven Parker
231,169 PointsYou might start by setting breakpoints as I suggested in my original answer.
Unsubscribed User
1,653 PointsUnsubscribed User
1,653 PointsI assume you want me to set a breakpoints for: transform.position = player.position + offset;
If so what would the condition be.
Steven Parker
231,169 PointsSteven Parker
231,169 PointsYou might want to move this question to the
Game Development
topic, Practiced Unity developers might recognize the cause of this from prior experience.Unsubscribed User
1,653 PointsUnsubscribed User
1,653 PointsWill do.
Steven Parker
231,169 PointsSteven Parker
231,169 PointsIt looks like you made a new one. I was thinking you might just change the category on this one using "edit question". But either way, remember to fix the code formatting!