Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial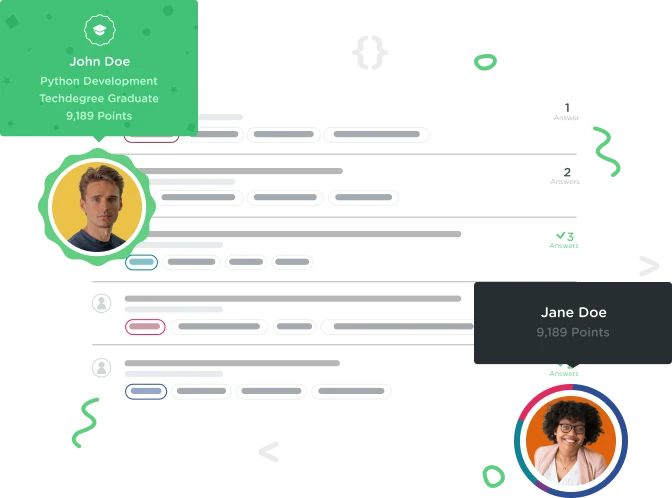

Adam Williams
4,215 PointsNearly have the extra credit solution. One little bug I could use some help with?
So I have solved the ability to print multiple reports when you add a student with the same name to the students array.
I did this by changing the print() method from innerHTML to insertAdjacentHTML so it wasn't simply overwriting the previous student.
I've nearly solved the student not found alert by using a variable called 'found' as a flag. It defaults to false and when a name is found updates to true.
This allows me to set an alert to run when 'false'. This works fine but intermittently it will throw up the alert for names that are included (yet still continues to print the correct report for that student).
Can anybody take a look and give me a clue please?
var message = '';
var student;
var search;
var found;
function print(message) {
// document.write(message);
var outputDiv = document.getElementById('output');
outputDiv.insertAdjacentHTML('beforeend', message);
}
function getStudentReport(student) {
var report = '<h2>Student: ' + student.name + '</h2>';
report += '<p>Track: ' + student.track + '</p>';
report += '<p>Points: ' + student.points + '</p>';
report += '<p>Achievements: ' + student.achievements + '</p>';
return report;
}
while (true) {
search = prompt("Enter a students name or type quit to end");
if (search === null || search.toLowerCase() === 'quit') {
break;
}
for (var i = 0; i < students.length; i += 1) {
student = students[i];
found = false;
if (student.name === search) {
message = getStudentReport(student);
print(message);
found = true;
}
} if (found === false) {
alert("There is no student with the name " + search + ".");
}
}
2 Answers
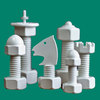
Steven Parker
229,732 Points
Preset "found" to false before the loop, not inside it.
Othewise, unless the search is for the last student listed, it will be false after the loop!
Also, there's never a need to compare a boolean variable to a constant — just test it directly. So instead of:
if (found === false)
Do this:
if (!found) // "if not found"

Erik Nuber
20,629 Pointswhile (true) {
search = prompt('Search student records: type a name (or type "quit" to end)');
search = search.toLowerCase();
var notInList = false;
if (search === "quit" || search === null) {
break;
}
for (var i = 0; i < students.length; i++) {
student = students[i];
if (student.name.toLowerCase() === search) {
listOfStudents.push(i);
notInList = true;
}
if ((i + 1) == students.length) {
message = getStudentReport(listOfStudents);
print(message);
}
}
if (!notInList) {
message = ("<p> The student " + search + " is not on the list.</p>");
print(message);
}
}
You are pretty close. Here is how I accomplished it using the same idea of a boolean value for if student wasn't found.
This was also how I dealt with multiple names being found. I created an array and stored the indexes so i could print it out.
You could use the indexOf() method as well that checks to see if the name exists inside the original array. if it returns a -1 you will know that the student doesn't exist and return the message too. Just a different way of doing it without a boolean value.

Adam Williams
4,215 PointsThanks. I'm going to have another crack at this using the indexOf() method. I completely forgot about that!
Adam Williams
4,215 PointsAdam Williams
4,215 PointsThanks, I'll get that amended :)