Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial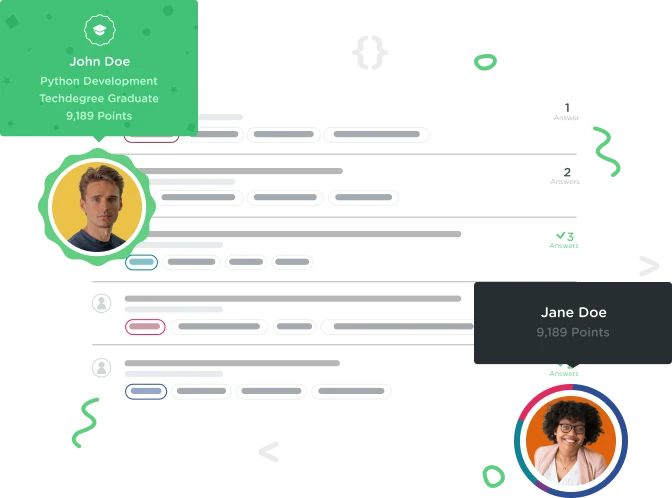

Maiia Spivak
3,038 PointsNeed a hint! How to approach this one?
Question:
I need you to create a new function for me. This one will be named sillycase and it'll take a single string as an argument. sillycase should return the same string but the first half should be lowercased and the second half should be uppercased. For example, with the string "Treehouse", sillycase would return "treeHOUSE". Don't worry about rounding your halves.
def sillycase(num):
return num = num.join(num[
9 Answers
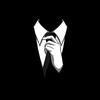
Jorge Corral
3,713 Pointsdef sillycase(string):
half = round(len(string)/2)
first_half = string[:half].lower()
last_half = string[half:].upper()
return first_half + last_half

Jared Azriel
6,228 Pointsdef sillycase(string):
stringlower = string[:(len(string)//2)].lower()
stringupper = string[(len(string)//2):].upper()
return (stringlower + stringupper)

Jared Azriel
6,228 PointsThis seemed to work perfectly fine for me. I thought it wasn't too bad.
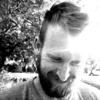
Jerehme Gayle
5,206 Pointsdef sillycase(string):
half = len(string) // 2
first_half = string[:half].lower()
last_half = string[half:].upper()
return first_half + last_half
This worked for me, it is very similar to Jorge Corral's solution the only difference is where he used the round() function I used // floor division to get half.
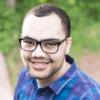
Philip Gales
15,193 PointsThey want you to lowercase the first half, and then uppercase the second half.
To do this you will need to know the length of the string (num). You will then need to somehow use that length to apply the lowercase function to that many first letters. Repeat this for the second half. I would maybe try using slices since we just learned that in the previous videos.
I would always advise not returning something immediately. Use multiple lines to write your code and then clean it up when it works.

Dean Vollebregt
24,583 Pointsdef sillycase(string):
mid = int(len(string)/2)
result = (string[:mid]).lower() + (string[mid:]).upper()
return result
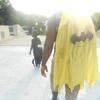
Prince Vonleh
Courses Plus Student 4,552 Pointsdef sillycase(a): b= int(len(a)//2) c= a[:b].lower() d= a[b:].upper() return c+ d

Peter Rzepka
4,118 Pointsstring = "treehouse"
# set string input to be "treehouse"
def sillycase(string):
half = round(len(string)/2)
# preset a variable half to equal the rounded length of input divided by 2
first_half = string[:half].lower()
# preset variable first_half equal to input first half lowered
last_half = string[half:].upper()
# perset variable last_half equal to input last half uppered
result = first_half + last_half
# set a new variable result equal to the concatenation of both
return result
# return the variable result out side function
sillycase(string)
# call to function
print(sillycase(string))
# print the function to screen
this is a full workable answer in Pycharm. Thanks Jorge Corral, I liked your answer
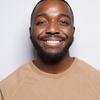
Jonathan Peters
8,672 PointsThank you for this explanation, this really helped!

Jassim Alhatem
20,883 PointsPeter Rzepka, I have a question, why did you do that:
first_half = string[:half].lower()
why the 'string' at the beginning of the slice?
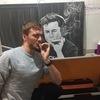
Tobias Mahnert
89,414 PointsUpdate your code guys,
my function is perfection but did not let me pass
def sillycase(s):
return s[:(len(s)/2)].lower() + s[(len(s)/2):].upper()

greyskymedia
Courses Plus Student 3,948 PointsI had similar. If you just replace the / with // in the above, it works.
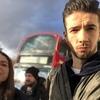
Jan Durcak
12,662 Pointsdef sillycase(text):
a = text.lower()[:len(text)//2]
b =text.upper()[len(text)//2:] + a
return b
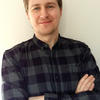
Arturas Budreika
Python Development Techdegree Student 5,464 PointsNice one! :)
but it should be:
def sillycase(text): a = text.lower()[:len(text)//2] b = a+ text.upper()[len(text)//2:]
return b
Thanks anyway!