Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial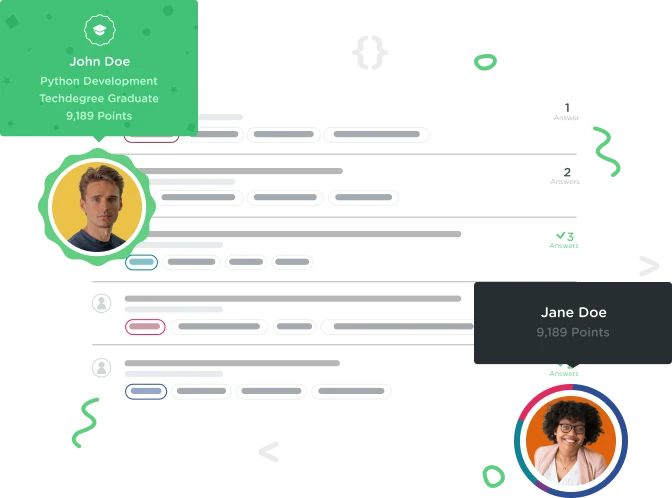

O'Ryan Alexander
Full Stack JavaScript Techdegree Student 5,671 PointsNeed a some help
Not understanding what this mean "Bummer! In the body of switchColor, change the color of the lamp to the value provided as an argument"
// Declare protocol here
protocol ColorSwitchable {
func switchColor(_ color: Color)
}
enum LightState {
case on, off
}
enum Color {
case rgb(Double, Double, Double, Double)
case hsb(Double, Double, Double, Double)
}
class WifiLamp: ColorSwitchable {
let state: LightState
var color: Color
init() {
self.state = .on
self.color = .rgb(0,0,0,0)
}
func switchColor(_ color: Color) {
_ = Color.rgb(1,1,1,1)
}
}
2 Answers

Thomas Dobson
7,511 PointsO'Ryan,
Your close, in your method assign self.color to the Color.rbg().
// Declare protocol here
protocol ColorSwitchable {
func switchColor(_ color: Color)
}
enum LightState {
case on, off
}
enum Color {
case rgb(Double, Double, Double, Double)
case hsb(Double, Double, Double, Double)
}
class WifiLamp: ColorSwitchable {
let state: LightState
var color: Color
init() {
self.state = .on
self.color = .rgb(0,0,0,0)
}
func switchColor(_ color: Color) {
self.color = Color.rgb(1,1,1,1) //doing it this way sets your lamp color to rbg(1,1,1,1). You will pass the code challenge but wont actually be able to set the color later.
}
}
instead try setting up your switchColor like I did. Under the class setup can see how it works with the testLamp variable I setup:
// Declare protocol here
protocol ColorSwitchable {
func switchColor(_ color: Color)
}
enum LightState {
case on, off
}
enum Color {
case rgb(Double, Double, Double, Double)
case hsb(Double, Double, Double, Double)
}
class WifiLamp: ColorSwitchable {
let state: LightState
var color: Color
init() {
self.state = .on
self.color = .rgb(0,0,0,0)
}
func switchColor(_ color: Color) {
self.color = color
}
}
var testLamp = WifiLamp() //lamp setup
testLamp.switchColor(Color.rgb(1, 1, 1, 1)) //change the lamp color
testLamp.color //retunrs rgb(1.0, 1.0, 1.0, 1.0)
I hope this helps.

O'Ryan Alexander
Full Stack JavaScript Techdegree Student 5,671 PointsThank you very much for your help