Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial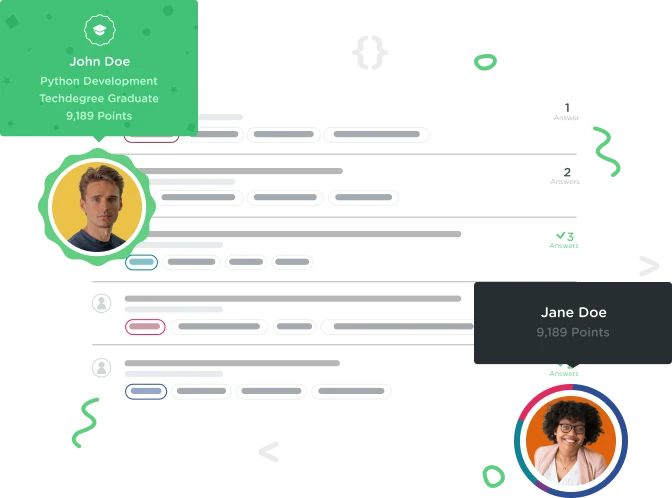
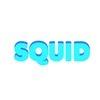
Alejandro Byrne
2,562 PointsNeed answer with explanation.. been stuck on this challenge for a few days..
Ok, could someone please tell me how to solve this? I think I'm pretty close. sorry to bug you...
def disemvowel(word):
word = list(word)
vowels = ['a', 'A', 'e', 'E', 'u', 'U', 'i', 'I', 'o', 'O']
for item in word:
if item in vowels:
word.remove(item)
else:
continue
return word
3 Answers
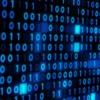
Alexander Davison
65,469 Pointsdef disemvowel(word):
result = []
for letter in word:
if letter.lower() not in 'aeiou':
result.append(letter)
return ''.join(result)
Step-by-step Explanation:
- We define the function
- We create an empty list containing no elements
- We go through every letter in the passed-in argument
- If the letter we're currently on lowercased isn't in 'aeiou'... (yes, you can check and see if a string is inside another string)
- (If the letter we are on isn't a vowel...) We add the letter to the
reuslt
list. - Finally, we join together the list by using
''.join(result)
and returning it. Think of''.join(result)
asresult.join('')
. It makes a little more sense that way, but remember that isn't valid Python!
I hope this helps
~Alex
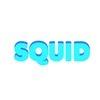
Alejandro Byrne
2,562 PointsAlright, thanks. It worked. Sorry for all the ruckus, I have to learn more about the .join method and checking wether a list/string is in another string/list.
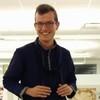
slavster
1,551 PointsAlexander Davison I really like your method of dealing with capitals. I was trying to write 2 For loops using the .lower() and .upper() function to handle both kinds. Your approach of looking at the word object, comparing all the letters in a single case, but then outputting the original case is much quicker. Very clever!
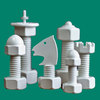
Steven Parker
230,274 PointsNever try to modify an item within a loop that is iterating that same item.
It will cause your loop to skip over some of the items. So instead, use a copy of the item to control the loop. An easy way to make a copy is using a slice with empty arguments.
Also, remember to convert your list back into a string before you return it!
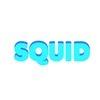
Alejandro Byrne
2,562 PointsHow's this?
def disemvowel(word):
word = []
vowels = ['a', 'A', 'e', 'E', 'u', 'U', 'i', 'I', 'o', 'O']
for item in word:
if item in vowels:
continue
else:
word.append(item)
word = str(word)
return word
[MOD: added ```python formatting -cf]
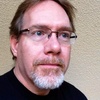
Chris Freeman
Treehouse Moderator 68,423 PointsExactly! By adding slice notations [:] a copy is made for the iterable which is then protected from changes to the actual value of word
:
for item in word[:]:
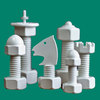
Steven Parker
230,274 PointsAlmost....
Applying my suggestions to your original code would give you this (and pass the challenge):
def disemvowel(word):
word = list(word)
vowels = ['a', 'A', 'e', 'E', 'u', 'U', 'i', 'I', 'o', 'O']
for item in word[:]: # loop using copy
if item in vowels:
word.remove(item)
else:
continue
return ''.join(word) # convert list back to string
But just for fun, Python also makes it possible to do essentially the same job in a single line:
def disemvowel(word):
return ''.join([letter for letter in word if letter.lower() not in 'aeiou'])
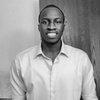
Modou Sawo
13,141 PointsHi Alejandro,
1) Try putting your vowels variable outside the function.
2) Inside the function, assign an empty list to a variable (i.e. output) 3) Create a for loop, like so:
for letter in list(word):
if not letter.lower() in vowels:
output += letter
output = ''.join(output)
return output
Like Steven said - you have to convert output list into a string.
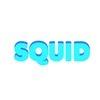
Alejandro Byrne
2,562 PointsHmmmm... I'm sorry. I don't understand what to do, what changes do I make to the original function? I don't understand the code you gave me. Sorry for being so stubborn.
Alexander Davison
65,469 PointsAlexander Davison
65,469 PointsTagging Chris Freeman