Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial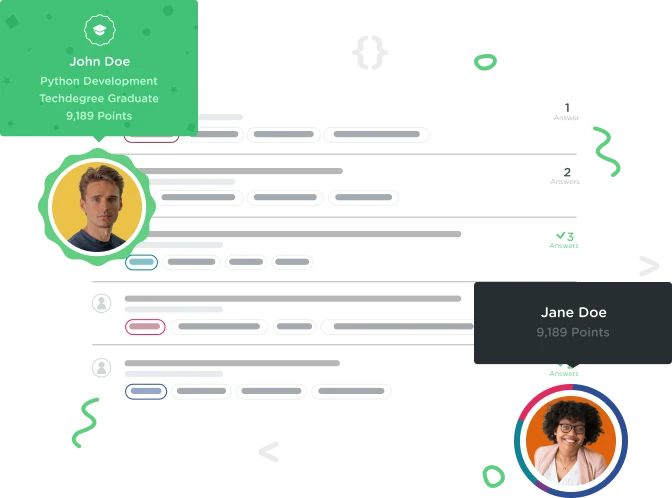

Mark Bradshaw
Courses Plus Student 7,658 PointsNeed assistance with a javascript quiz question
Here's the question:
create a function named 'arrayCounter' that takes in a parameter which is an array. The function must return the length of an array passed in or 0 if a 'string', 'number' or 'undefined' value is passed in.

Mark Bradshaw
Courses Plus Student 7,658 PointsSorry Gareth, I feel like I've tried everything. Here's what I setup so far:
function arrayCounter ([1, 2, 3]) {}
1 Answer

Gareth Borcherds
9,372 PointsOkay, so far you are correct, I would just make the array a variable rather than an actual array. So we start with
function arrayCounter (array) {
}
Now, the next step is that we need to return the length of the array. Do you know how to get the length of an array?

Mark Bradshaw
Courses Plus Student 7,658 PointsI do. Inside of the curly braces I would add console.log(array.length).

Gareth Borcherds
9,372 PointsOkay, so that would log it to the console. To actually return the value you would use return array.length;
So now our code looks like this:
function arrayCounter (array) {
return array.length;
}
Now however, we don't know if the variable passed in as array is actually an array. We need to add a check to see if the variable is a string, number, or undefined. So we need an if statement. Do you know how we check the type of variable something may be and put it in an if statement? Once we get that, we'll be complete.

Mark Bradshaw
Courses Plus Student 7,658 PointsThis is where I'm lost in this process. Can you explain how the if statement would be applied here? I know that it would go within the curly braces but I don't know what should be equal to what.

Gareth Borcherds
9,372 PointsSo we are going to use the function typeof and put it in the if statement. If our statement returns true then we will return 0, else we'll return the length.
function arrayCounter (array) {
if ( typeof array === 'string' || typeof array === 'number' || typeof array === 'undefined') {
return 0;
}
return array.length;
}
Does that make sense?
Gareth Borcherds
9,372 PointsGareth Borcherds
9,372 PointsBefore just giving you the answer, what are some code things you have tried? Where have you gotten with trying to do this? It's always best if we can help you edit code you've tried rather than giving out answers.