Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial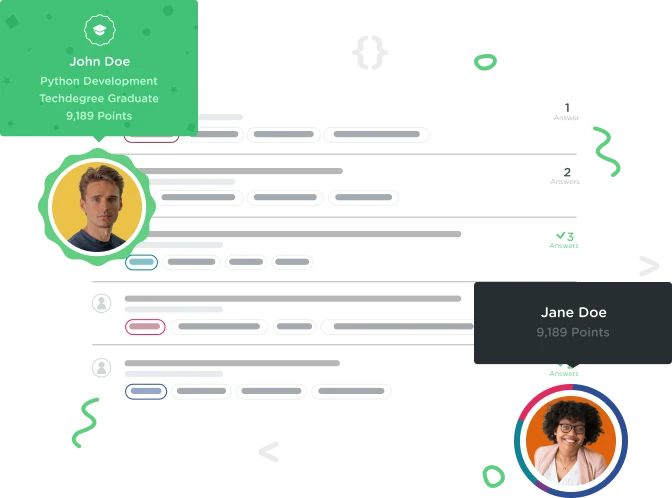

Jonathan Beard
1,681 PointsNeed assistance with dir_contains function
I have been working on this challenge without any luck. It is asking to verify the existence of files in a list. I think this does that but I'm sure I'm missing something.
The error I get is some returned False. I wish you could debug on the console to get better information.
def dir_contains(path, file_list):
for files in file_list:
if os.path.isfile(files):
return True
else:
return False
5 Answers
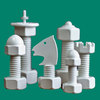
Steven Parker
230,274 PointsYou seem to have a good idea there, and you're pretty close. Here's a few hints:
- you must join the path with each file name to be able to check it
- you must check every file before you can return "True"
- you can return "False" as soon as one file doesn't check out

Jonathan Beard
1,681 PointsI'm just stuck on the checking all before returning True....
def dir_contains(path, file_names):
for files in file_names:
file = os.path.join(path, files)
if os.path.isfile(file):
return True
else:
return False
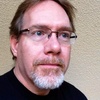
Chris Freeman
Treehouse Moderator 68,423 PointsYou have the right idea, but there are two issues:
- you are returning in the first iteration whether it's True of False. Instead, only return when you known one is False or all are True.
- the argument to
isfile
needs to be a full path. Useis.path.join
to create full path from function parameters.
Post back if you need more help. Good luck!!!

Jonathan Beard
1,681 PointsThis seems to have worked. However, is this the best way to get the correct answer?
def dir_contains(path, file_names):
true_files = 0
false_files = 0
for files in file_names:
file = os.path.join(path, files)
if os.path.isfile(file):
true_files += 1
else:
false_files += 1
if true_files == len(file_names):
return True
else:
return False
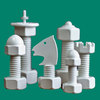
Steven Parker
230,274 PointsYou didn't really need to count the files, you can return "False" the first time one isn't found, and then return "True" after the loop has finished.

Jonathan Beard
1,681 PointsI had some one help me here at work.
def dir_contains(path, file_names):
for files in file_names:
file = os.path.join(path, files)
if not os.path.isfile(file):
return False
return True
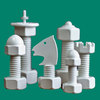
Steven Parker
230,274 PointsWith a handy private mentor, you may not need the forum! But that's exactly what I had in mind when I gave those hints originally.