Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial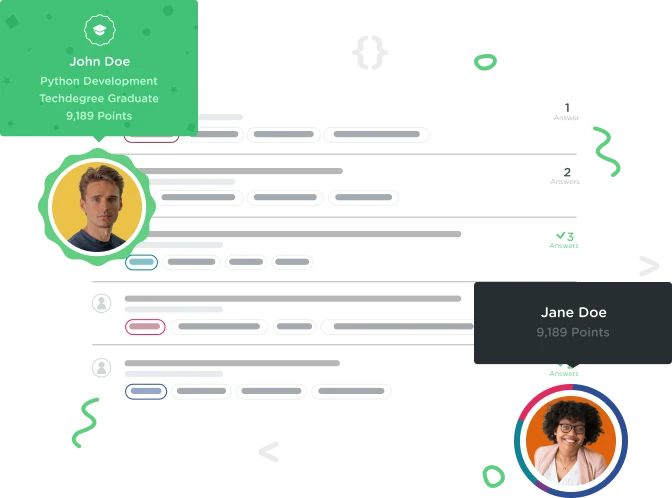
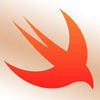
Jeff McDivitt
23,970 PointsNeed assistance with Swift generics
Since we don't want to interact with the array directly we need to add some code to let users know if the underlying data store contains elements. For this task add a computed property: isEmpty of type Bool that lets us know whether the queue is empty.
struct Queue<Element> {
var array: [Element]
}
5 Answers
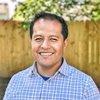
Helmut Granda
10,343 Pointspublic var isEmpty: Bool {
return array.isEmpty
}
It looks like you may encounter some bumps along the way, I recommend reading this article which seems that is what this challenge is based on:
https://www.raywenderlich.com/148141/swift-algorithm-club-swift-queue-data-structure

bobpeterson
Courses Plus Student 4,264 PointsHi Jeff,
You need to initialize your variable stored property to an empty array before your computed property, isEmpty, will work:
var array: [Element] = []
Now you should be able to solve the second challenge.
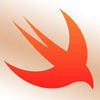
Jeff McDivitt
23,970 PointsYes Sir - Here is how I completed it
mutating func dequeue() -> Element? {
if self.array.isEmpty == false {
return self.array.remove(at: 0)
} else {
return nil
}
}
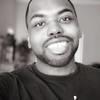
Alphonso Sensley II
8,514 PointsAwesome! thank you!
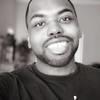
Alphonso Sensley II
8,514 PointsHi Jeff did you ever pass task 5/5 on this challenge? Im a little stuck as well.

harshil bhatt
Courses Plus Student 2,722 Pointsstruct Queue<Element> {
var array: [Element] = []
public var isEmpty: Bool {
return array.isEmpty
}
var count : Int {
return array.count
}
mutating func enqueue(_ Value : Element) {
array.append(Value)
}
mutating func dequeue() -> Element? {
if self.array.isEmpty == false {
return array.remove(at : 0)
}
else {
return nil
}
}}
// HOPE ITS useful i have done this successfully

harshil bhatt
Courses Plus Student 2,722 Pointsstruct Queue<Element> {
var array: [Element] = []
public var isEmpty: Bool {
return array.isEmpty
}
var count : Int {
return array.count
}
mutating func enqueue(_ Value : Element) {
array.append(Value)
}
mutating func dequeue() -> Element? {
if self.array.isEmpty == false {
return array.remove(at : 0)
}
else {
return nil
}
}}
Jeff McDivitt
23,970 PointsJeff McDivitt
23,970 PointsI appreciate the assistance, but I have had this before and it does not complete the challenge