Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial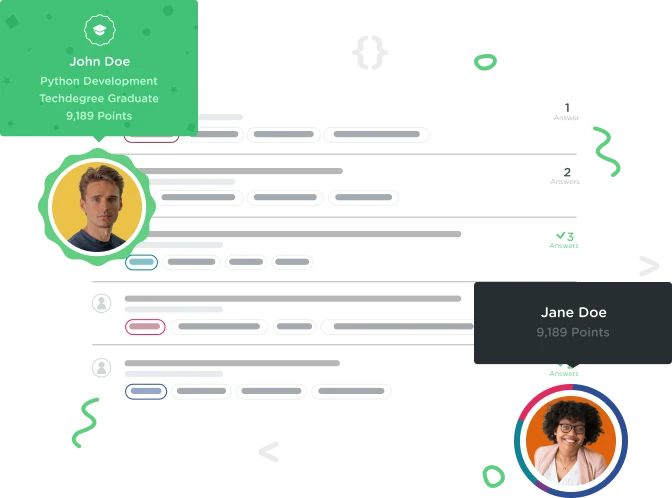

Jonathan Adams
544 Pointsneed code help
Hello I am stuck on this guy. I guess I really dont know what Craig is asking us to do and I am unable to make the comparison from the lesson example to this question since while similar are two different questions with different answers.
The question is asking for us to use a method signature, add a new method called drive that is different than the one he already made, that performs 1 lap and takes no argument.
In my code below I tried to figure it out. I created a new method that I thought was what he wanted which also followed the 5 minute video before. So I made a second public void drive method.
I know we need to set a default value by passing in 1, but I am not sure for what variable. Additionally when I try to write out "laps++"; which to me should take the laps value and add 1, it doesnt work and says "can not find symbol" and is pointing to my laps++; line.
I am not sure what I am doing wrong since in the above method laps was listed. We know that mBarsCount = current mBarsCount - laps
So I am trying to add +1 to the laps variable, but apparently it doesnt exist? How can I solve? what am I missing?
public class GoKart {
public static final int MAX_BARS = 8;
private String mColor;
private int mBarsCount;
public GoKart(String color) {
mColor = color;
mBarsCount = 0;
}
public String getColor() {
return mColor;
}
public void drive(int laps) {
// Other driving code omitted for clarity purposes
mBarsCount -= laps;
}
public int drive() {
laps++;
}
public void charge() {
while (!isFullyCharged()) {
mBarsCount++;
}
}
public boolean isBatteryEmpty() {
return mBarsCount == 0;
}
public boolean isFullyCharged() {
return mBarsCount == MAX_BARS;
}
}
3 Answers
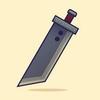
Allan Clark
10,810 PointsNo this method should be in addition to the existing drive method. He actually meant overload instead of override, overloading is when you have multiple methods in the same class with the same name, but they have different signatures (that is to say the parameters are different).
drive is a method not a variable so we cannot say drive = 1. When we have drive(1); we are calling the existing drive method that will handle what should happen to the Object.
public void drive(int laps) {
// Other driving code omitted for clarity purposes
mBarsCount -= laps;
}
public void drive() { // when this method is called we then call the existing drive method that accepts
drive(1); // a parameter and we pass it 1
}
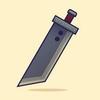
Allan Clark
10,810 PointsThe variable "laps" is not within the scope of the new drive() method. That variable is the the placeholder for what is accepted in the previous drive method. What Craig wants you to do is to make an overloaded method such that, if the method call drive() is made, with no parameters, it will function like driving only one lap. Also, we don't need a return here, this method just changes the Object's internal state. It also wants you to use the existing drive method inside your new method. So it should look something like this:
public void drive() {
drive(1);
}
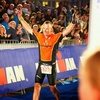
Steve Hunter
57,712 PointsHiya,
There's an existing method called drive
that takes the number of laps the kart should drive.
In this challenge, you want to override that with a new method signature that takes no parameters.
The question asks you to create a method signature for drive
that takes no parameters and makes the kart do one lap. You can use the existing method within this new one. The new method looks something like this:
public void drive() { // new method with no parameters
drive(1); // calls the existing method for just one lap
}
I hope that makes sense!
Steve.
P.S. I'm off out now, but please shout back if that's not clear. I'll walk you through it step-by-step. Post any questions back here and I'll get back to you when I'm back home.

Jonathan Adams
544 PointsSo to confirm what you are saying. Craig is asking us to essentially remove the existing drive method and replace it with one similar to yours? Is that what you mean by override?
Additionally could you explain the drive(1); line to me? This looks different than what I am used to.
typically when assigning a variable I would write like drive = 1. Can you explain to me what that line is doing and why it is that way and not =1? I understand we are assigning a default value, I just don't understand the layout. Maybe I am missing it completely.
Jonathan Adams
544 PointsJonathan Adams
544 PointsOk so just so I understand. if we did drive(2). We should expect it to run the public void drive (int laps) method twice?
Allan Clark
10,810 PointsAllan Clark
10,810 PointsNegatory, it would call the existing method only once but pass it the parameter '2'. It would not touch the method we created at all (i.e. the drive() method).
Allan Clark
10,810 PointsAllan Clark
10,810 PointsThat is the point of the overload, if the drive call has a parameter the JVM will use public void drive(int laps) method, if the drive call has no parameter it will use the one we created, public void drive().