Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial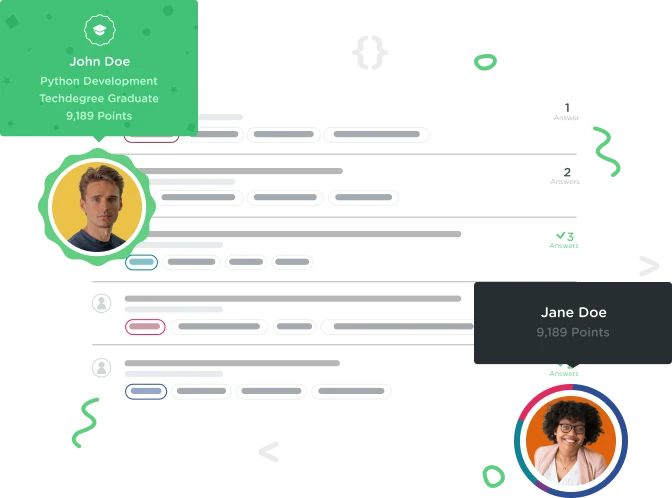
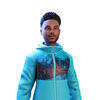
Calvin Secrest
24,815 PointsNeed help
Confused about how to Change SequenceDetector.Scan to an abstract method.
namespace Treehouse.CodeChallenges
{
public class RepeatDetector : SequenceDetector
{
public override string Description => "Detects repetitions";
public override bool Scan(int[] sequence)
{
LastScannedSequence = sequence;
if(sequence.Length < 2)
{
return false;
}
for(int i = 1; i < sequence.Length; ++i)
{
if(sequence[i] == sequence[i-1])
{
return true;
}
}
return false;
}
}
}
namespace Treehouse.CodeChallenges
{
public abstract class SequenceDetector
{
public virtual string Description => "";
public int[] LastScannedSequence { get; protected set; }
public virtual bool Scan(int[] sequence)
{
LastScannedSequence = sequence;
return true;
}
}
}
1 Answer

Brian DuPont
30,448 PointsIn the SequenceDetector class, you need to change virtual to abstract, and also get rid of the method body. So it would be just like this: public abstract bool Scan(int[] sequence); We want the class inheriting this method to override and implement the method with a method body.