Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial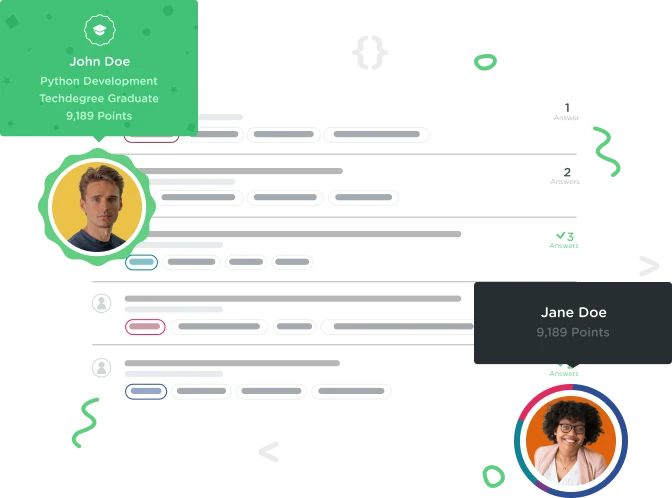

Tian Siang Ong
5,911 PointsNeed Help
Getting all sorts of errors. :( Can someone assist to point out my mistake please? thanks
class BankAccount attr_reader :name
def initialize(name) @name = name @transactions = [] add_transaction("Beginning Balance", 0) end
def credit(description, amount) add_transaction(description, amount) endss end
def debit(description, amount) add_transaction(description, -amount)
def add_transaction(desscroption, amount) @transactions.push(description: description, amount: amount) end
def balance balance = 0.0 @transactions.each do |transaction| balance += transaction[:amount] end return balance
def to_s "Name: #{name}, Balance: #{sprintf("%0.2f", balance)}" end
def print_register puts "#{name}'s Bank Account" puts "-" *40
puts "Description".ljust(30) + "Amount".rjust(10)
@transactions.each do |transaction|
puts transaction [:description].ljust(30) + sprintf("%0.2f", transaction[:amount]).rjust(10)
end
puts "-" *40
puts "Balance:".ljust(30) + sprintf("%0.2f", balance").rjust(10)
puts "-" *40
end
bank_account = BankAccount.new ("Jason") bank_account.credit("Paycheck", 100) bank_account.debit("Groceries", 40) bank_account.debit("Gas", 10.51) puts bank_account puts "register:" bank_account.print_register
1 Answer

David Thrower
6,693 PointsSometimes the code doesn't match here what you wrote, but from what I am seeing here:
One potential error that I see is that the method
Issue 1:
def initialize(name) @name = name @transactions = [] end 1. <------ This end is missing. You declared a method without telling Ruby where the method ends, so ruby is confused and thinks everything else is part of the method but is saving you from bigger trouble by counting the places where an end keyword is expected and stopping the app from running unless there is an end for each def or if or whatever else that expects an end...
Issue 2:
The method add_transaction you left off the def before it.
def add_transaction("Beginning Balance", 0) end # Issue 3, you did not include code in the method add_transaction to push a description and an amount into the array variable @transactions before the end keyword. Also you did add code to the method 'credit' which tells this method to process these values, but this cannot receive these values because of this and: Issue 4: You put quotation marks around beginning_balance. when you define a method like this: method_name(parameter_1, parameter_2), you are telling ruby to to expect a value to pass in as arguments via these two variables parameter_1, parameter_2. Instead, you have put a string and an integer here, where 2 variables should go.
Issue 5 the two arguments that we used when I did one like this were description and amount, which would make sense, because they are what the next method you wrote 'credit' will pass in when it calls add_transaction(description, amount).
Issue 6:
def credit(description, amount) add_transaction(description, amount) endss end # you wrote end twice and misspelled it one of the two times.
I have to stop here for now, but I would highly encourage you to start at the beginning and re-build this step by step with the exercises from the beginning of the module. You will get error checks along the way, and it is always easier to build a complex project on step at a time and address errors one at a time than it is to try to fix 100 errors at once, which confound each other and make it harder to find out what is wrong. Same as is is easier build a building with on brick at a time and check to make sure that the last brick you laid is correct than it is to build it all at once then have to find many errors all at once that are hard to find and hope you can figure out why the house is crooked before it collapses.