Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial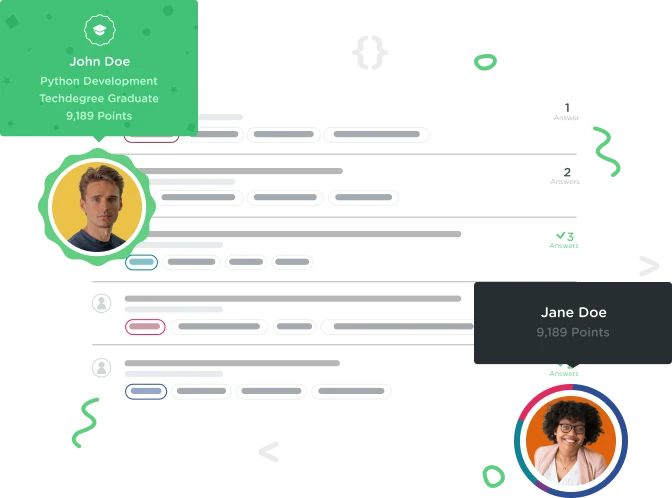
Raiyan Ahmad
3,622 Pointsneed help
Whats wrong
def disemvowel(word):
word.lower()
while True:
try:
word.remove('a', 'e', 'i', 'o', 'u')
except ValueError:
break
return word
2 Answers
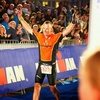
Steve Hunter
57,712 PointsRemoving vowels[i]
? Why? Looping over the len()
of vowels
? Why?
And, to complete this task, you are better off adding to an output list/string letters that aren't a vowel. i.e. not in vowels
. If you start removing from an iterable you are looping over, you get nasty consequences of skipping indexes.
Start by declaring your list of vowels. Create an empty string to output. Iterate over word
. At each iteration see if the letter is not in the list of vowels. If not, add to output. Else, carry on. Return the output string after the loop.
This looks like:
def disemvowel(word):
vowels = ['a', 'e', 'i', 'o', 'u']
output = ""
for letter in word:
if letter.lower() not in vowels:
output += letter
return output
About the for
loop issue; have a read of this which covers it off.
Steve.

Rich Zimmerman
24,063 PointsYou can only remove one item at a time this way with the remove() method. You can try looping through a list of the letters you want to remove. Or loop through the letters in the word and checking if the letter is in the list of vowels and remove it.

Rich Zimmerman
24,063 PointsSomething like:
def disemvowel(word):
vowels = ['a', 'e', 'i', 'o', 'u']
word = word.lower()
for i in range(0, len(vowels)):
word.remove(vowels[i])
return word
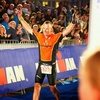
Steve Hunter
57,712 Points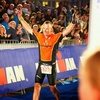
Steve Hunter
57,712 Pointsword
should be returned minus vowels only. Not lowercased as well.
So, add uppercased vowels to your list and remove the .lower()
line completely.
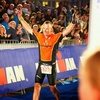
Steve Hunter
57,712 PointsDidn't work for me - but that's probably just me ... it's late here now.
def disemvowel(word):
vowels = ['a', 'e', 'i', 'o', 'u', 'A', 'E', 'I', 'O', 'U']
#word = word.lower()
for i in range(0, len(vowels)): # i tried with `- 1` too
word.remove(vowels[i])
return word
Rich Zimmerman
24,063 PointsRich Zimmerman
24,063 PointsThe remove method removes from the string you're calling it on, if I was looping over the string and removing from it, you would be correct. That would cause problems.
In this case I was looping over the list of vowels.
Your method is cleaner, yes, but the code challenge comes with
return word
Already supplied, so the beginner python developer may not think to create an output string and return that instead, I was trying to keep that in mind with my one, of many, possible ways of solving this challenge.
Steve Hunter
57,712 PointsSteve Hunter
57,712 PointsHi Rich,
I see your point but your code doesn't work. I think that's because you've amended the input
word
to be lowercase but the output should retain the input case.My code could equally assign
output
toword
at the end and then return that but that's trite, at best. The challenge doesn't define the return ofword
as compulsory. But, I can see where you're coming from there.And, yes, there are a number of ways of solving this - I chose the clearest code-wise. There's a load of geeky ways of doing that which really should never be used! (And which are way beyond my intellect!)
Steve.