Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial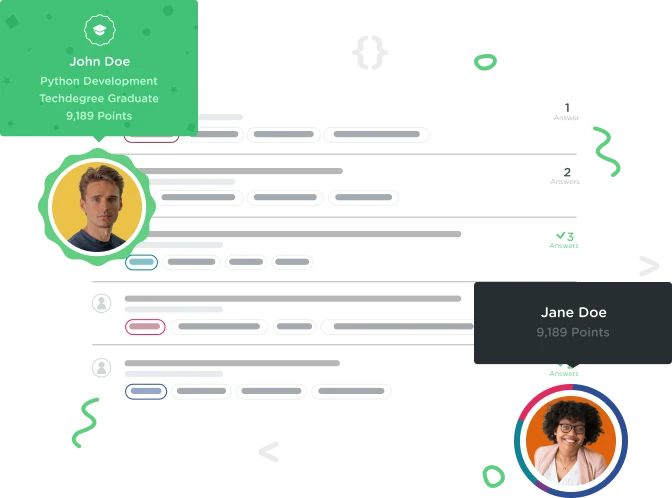

shihab solaman
211 Pointsneed help
pls help
def combiner(a):
2 Answers
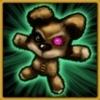
Dave Harker
Courses Plus Student 15,510 PointsHi shihab solaman,
Just going to work through this one with you ...
Create a function named
combiner
that takes a single argument (we're told later it's a list)
def combiner(someList):
which will be a list made up of strings and numbers. (example given: ["apple", 5.2, "dog", 8])
This will be passed in the testing ... just take note that multiple number types can be passed in ... i.e. int and float
We know we'll need some temporary variables, one for string values and one for number values
myString = '' # initializing with empty string
sumOfNums = 0 # initializing with a 0 value
Return a single string that is a
combination
ofall of the strings
in the list and then thesum of all of the numbers
. (outcome to example list given: appledog13.2)
So we know we're going to need to iterate through the passed in list
for entry in someList:
Be sure to use isinstance to solve this as I might try to trick you.
Now with each entry we need to determine if it's a string, int, or float. Let's use a couple of if statements, you can do this several ways, this is just the way I'm going to demo it though.
if isinstance(entry, str):
myString += entry
if isinstance(entry, (int, float)): # of note here is that we're checking the type against both int AND float
sumOfNums += entry
Now that we have all the strings joined together, and all the number values added up, let's join them together and return it as a single string:
myString += str(sumOfNums)
return myString
And ... we're done
I hope this breakdown helps you out.
Please take a moment to think about each part we've gone through above (rather than just copy/paste the code).
OK, so if you've followed along you should now have something that looks like this:
def combiner(someList):
myString = ''
sumOfNums = 0
for entry in someList:
if isinstance(entry, str):
myString += entry
if isinstance(entry, (int, float, complex)):
sumOfNums += entry
myString += str(sumOfNums)
return myString
Best of luck, and happy coding,
Dave
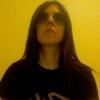
jopapadaki
7,311 PointsThanks Dave,
Btw I noticed that if you forget to add float on second if, you get an error message.