Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial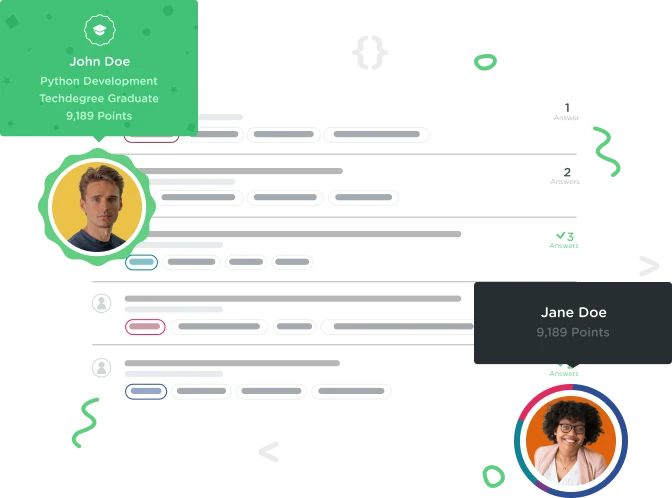
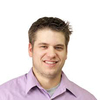
Kevin Korte
28,148 PointsNeed help building my Active Record schema
So I need help building out my schema for a rails app.
In one table, I'll store parts. I am considering using Single Table Inheritance here, since for parts I could have oil filters, air filters, spark plugs, etc, all which would have a brand, part_number, and type.
I'd also have a table of machines, most likely a title like Year Make Model.
Each part has many machines, and each machine has many parts. It would seem I would want to use a has many through relationship with a join table.
On the view, I need to be able to separate out the parts based on their type. So loop through and grab all air filters that are associated with that machine, than loop through and grab all oil filters etc.
I initially thought I wanted an array of part_id numbers for each type stored with the machine in the machine table, but that seems frowned upon.
Am I on the right track designing my schema? If all goes well, this would hold 10's of thousands of parts, and thousands of machines, and I need an easy way to tie a part number to a machine.
Reverse search would also be a feature I would want to look at; giving the user the option to enter a part number, and get a list of all machines that it is associated with.
1 Answer
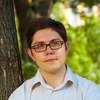
Artem Prytkov
11,932 PointsI think your idea is generally right: just dont forget to make indexes on bridge table and use "include" method in ActiveRecord in order to avoid n+1 queries. Not so sure about STI - it
s useful when you have models with same data but different methods? but in your case all objects in table should be same.
It might be better to extract third table PartType where you will store types. This way you can easily get your part details with query like
PartType.where(type: "filter").parts
Kevin Korte
28,148 PointsKevin Korte
28,148 PointsThanks for that. I got it working today, using
has_many through
and I have afitments
table that is joining parts and machines together. I went this route because I believe I'll also be able to store a position integer and control the views output. I'm able to add and remove relationships in the console, so all is good so far.I created a migration, and added my index column on my fitments table. My migration is basic, and looks like this:
Here is my question, I'm still learning how this works, but is Rails smart enough to populate these columns for the data that was existing before the migration, and I assume it will populate the index's going forward. Is there anything more I have to do in my model or controller? And is it normal that I can't find these indexs in the console? I just wanted to see if they had values or not.
Appreciate the help.