Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial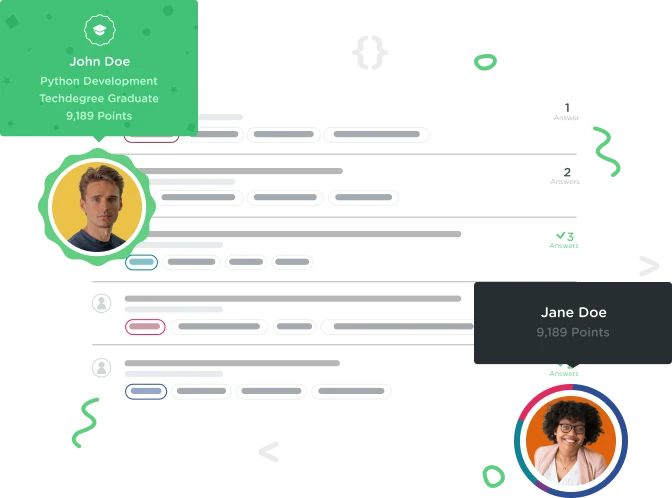

Nas Jones
7,849 PointsNeed help completing this challenge
Tried playing around with it and it wont work. I tried 'a.link' also
var inputValue = document.getElementById('linkName').value;
inputValue = document.getElementById('link').textContent;
<!DOCTYPE html>
<html>
<head>
<title>DOM Manipulation</title>
</head>
<link rel="stylesheet" href="style.css" />
<body>
<div id="content">
<label>Link Name:</label>
<input type="text" id="linkName">
<a id="link" href="https://teamtreehouse.com"></a>
</div>
<script src="app.js"></script>
</body>
</html>
1 Answer
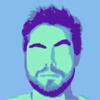
Cameron Childres
11,820 PointsHi Nas,
You're incredibly close -- the only issue is the order in your assignment on the second line.
The left side of the assignment operator is what a value will be assigned to, the right side is the value being assigned. To give document.getElementById('link').textContent
the value from inputValue
you just need to swap around the order:
document.getElementById('link').textContent = inputValue;
Nas Jones
7,849 PointsNas Jones
7,849 PointsThis helped thank you. I don't understand what's the difference between putting the variable on the other side, does it make that much of a difference?
Cameron Childres
11,820 PointsCameron Childres
11,820 PointsIt certainly does! You can think of the left side as receiving the value and the right side as giving the value.
As a little demonstration try this out in your console:
This won't work as it is trying to change the value of the number 4 to y (since y is on the right side).
If we switch it around then 4 is given to y, which works:
As another example, try to figure out what will be logged to the console here:
Nas Jones
7,849 PointsNas Jones
7,849 PointsI see, the left is for receiving the right is for giving. For the example you showed me on the bottom you wanted the variable x to show first so blue would show up first. Then below that red would show up. Where you confused me is "x=y" wouldn't that show an error? I'm confused as to how that works
Cameron Childres
11,820 PointsCameron Childres
11,820 PointsJust a bit of a brain teaser there. The "x=y" part is key: x is going to receive the value given to it by y. In other words, x is being reassigned to have the value of y.
Try the code out in your console to see the result or let me know if you'd like the answer.
Nas Jones
7,849 PointsNas Jones
7,849 PointsI just applied that code in the console and it was just like you said x is now y so both show "red" when i logged it in the console. I see now, thank you for the help!.