Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial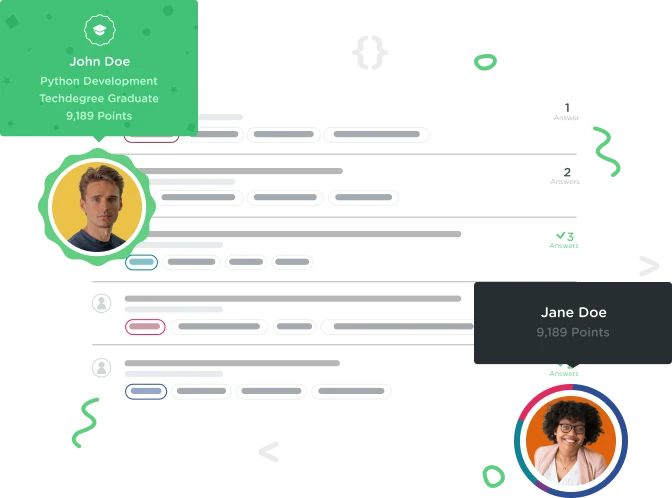

Matt Frerichs
4,015 PointsNeed help creating oddNumbers array in closures challenge.
Hi Everybody,
I am having difficulty creating the oddNumbers array in the second step of the following code challenge:
Given an array of integers, called numbers, use the filter function (and the helper function we created) to create a new array containing only odd numbers. Assign this new array to a constant named oddNumbers.
When the isOdd Function returns the boolean values, how do I convert them to Integer values for the new array? Does it automatically return integers because it filters the numbers array?
I need help completing this challenge! Thanks for the help.
let numbers = [Int](0...50)
// Enter your code below
func isOdd(i: Int) -> Bool {
return i % 3 == 0
}
let oddNumbers = numbers.filter(Int, isOdd)
3 Answers
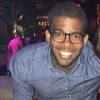
Gianni Guitteaud
7,648 PointsHey Matt, I think the problem came when you completed the first challenge wrong. The isOdd function should "return i % 2 != 0" instead of " return i % 3 == 0"
And to pass the last test, you need to pass in the function isOdd as a parameter to filter, therefore "Int" can be removed from your code.
func isOdd(i: Int) -> Bool {
return i % 2 != 0
}
let oddNumbers = numbers.filter(isOdd)
Should work now :D

Manya Sachdeva
568 Pointslet numbers = [10,423,802,765,943,12,405,230,1348,128,237] let oddValues = numbers.filter {$0%2 != 0}
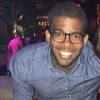
Gianni Guitteaud
7,648 PointsHi Matt, good to know it worked for you.
The reason I'm using "i % 2 != 0" is to say any number divided by 2 isn't equal to 0, which in other terms means it's an odd number. Using "i % 3 == 0 " may work for some odd numbers however it is not consistent across the entire array and will create mistakes. A quick example
let array = [1,2,3,4,5,6,7,8,9]
let filteredArray = array.filter { $0 % 3 == 0}
filteredArray // -> 3,6,9 (and number 1 is missing)
Check this link (https://developer.apple.com/library/prerelease/ios/documentation/General/Reference/SwiftStandardLibraryReference/Array.html) Very useful to understand the array function in Swift.

Matt Frerichs
4,015 PointsThanks, I'll brush up on that.
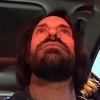
John DiPanfilo
Courses Plus Student 3,071 PointsI love your breakdown Gianni! Very helpful!
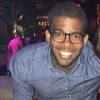
Gianni Guitteaud
7,648 PointsThanks for the feedback John DiPanfilo :) . Glad I could help. Have a good day

Scott Eremia-Roden
Courses Plus Student 15,623 PointsWhy not use
i % 2 > 0
Matt Frerichs
4,015 PointsMatt Frerichs
4,015 PointsThanks for the help Gianni!
Matt Frerichs
4,015 PointsMatt Frerichs
4,015 PointsDoes the Bang "!" operator unwrap the boolean values to return integers in the array?